A fictitious company Northwind Traders had a fair amount of success with the statically exposed product catalog that allows third parties to display the catalog on their own web sites. An exceptionally small amount of code is required to create a jQuery cross-domain static client of the back office web app created with Code On Time.
Northwind Traders have decided to offer search capabilities of the back office web app to their premium integration partners.
A possible implementation of a search client is shown next.
If a user clicks Find without entering a search sample, then the first one hundred products are retrieved in alphabetical order.
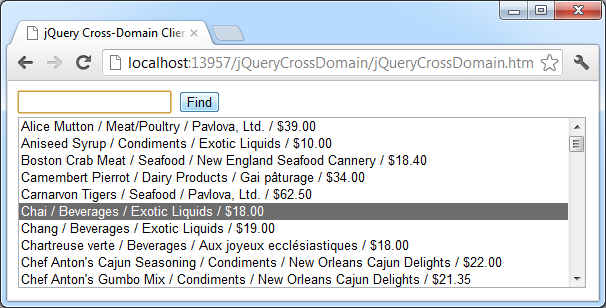
This is the result that matches “40 biscuit” search criteria.
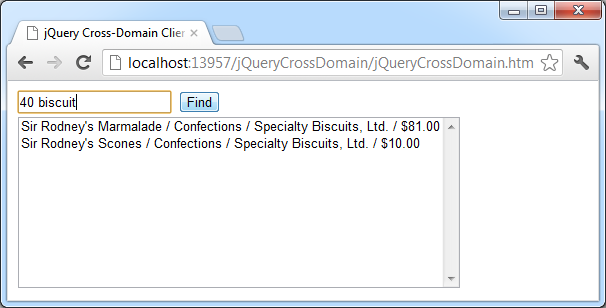
The back office web app at http://demo.codeontime.com/northwind/pages/products.aspx displays the same exact result as follows.
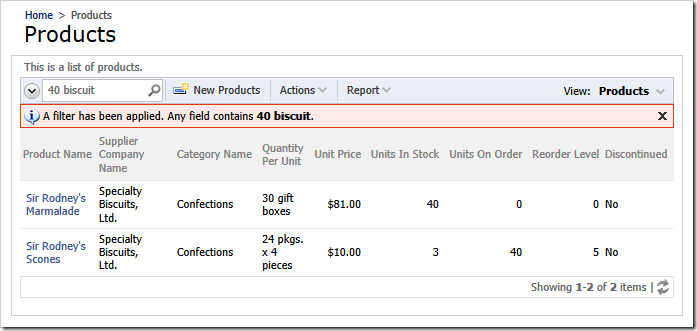
The HTML page implementing a sample search client works across domains in any web browser.
<!DOCTYPE html>
<html xmlns="http://www.w3.org/1999/xhtml">
<head>
<title>jQuery Cross-Domain Client</title>
<script src="http://ajax.googleapis.com/ajax/libs/jquery/1.8.1/jquery.min.js"></script>
<script type="text/javascript">
$(document).ready(function () {
$('#ProductListPanel').hide();
$('#Query').focus();
$('#FindButton').click(function (e) {
e.preventDefault();
var query = '?_sortExpression=ProductName&_q=' +
encodeURIComponent($('#Query').val());
$.ajax({
url: 'http://demo.codeontime.com/northwind/appservices/Products' + query,
cache: false,
dataType: 'jsonp',
success: function (data) {
$('#ProductList option').remove();
$.each(data.Products, function (index, product) {
$('<option>')
.text(
product.ProductName + ' / ' +
product.CategoryCategoryName + ' / ' +
product.SupplierCompanyName + ' / ' +
product.UnitPrice)
.attr('value', product.ProductID)
.appendTo($('#ProductList'));
});
$('#ProductListPanel').show();
}
});
});
});
</script>
</head>
<body>
<input id="Query" type="text" />
<button id="FindButton">
Find</button>
<div id="ProductListPanel">
<select id="ProductList" size="10">
</select>
</div>
</body>
</html>
The page statically links jQuery library from CDN provided by Google.
The search starts when a user clicks on the Find button.
The “click” handler declares a query variable that contains “_sortExpression” and “_q” parameters. The first parameter specifies the sort order of returned products. The search parameter “_q” is the sample for the Quick Find feature of the web app.
Next, the “click” handler invokes ajax method to retrieve data from the web app. The key parameters of the method are url and dataType. The first parameter defines the REST URI of Products data controller. The second parameter indicates that a cross-domain web request must be executed by jQuery library.
If the request is successful, then a list of products populates the list box.