Cloud On Time app runs cloud applications created with Code On Time products directly on your device with an optional offline mode. Integrated access to device camera and sensors contributes to enhanced user experience.
This user guide explains how to connect, install, run, and manage the front-ends of the connected cloud applications in Cloud On Time app.
The home screen of Cloud On Time app displays Connect button if there are no connected clouds.
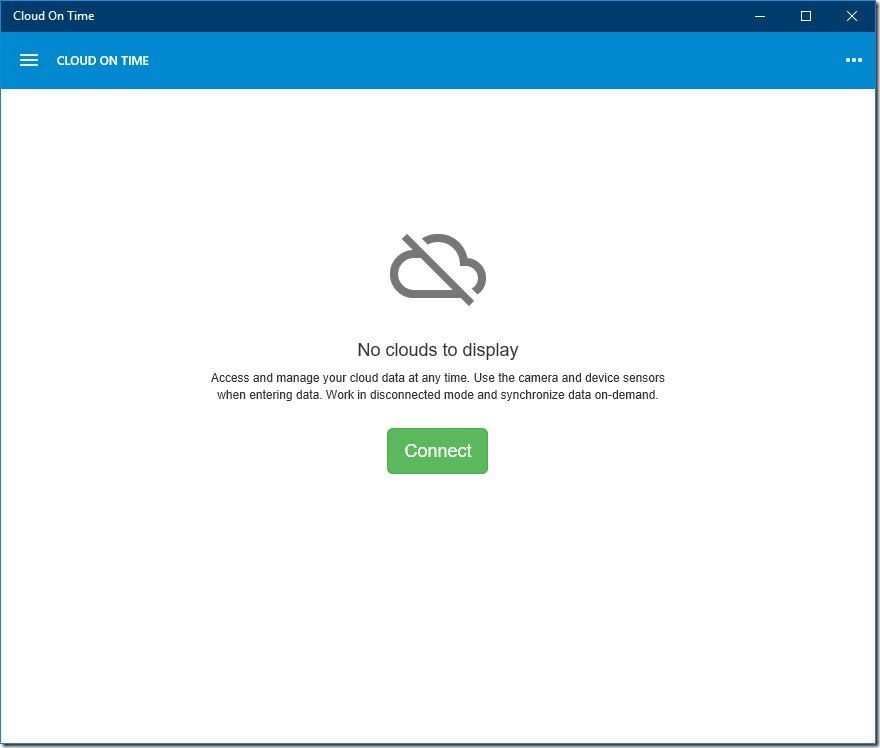
Tap “Connect” to connect to an application running in the cloud. When prompted, enter either the application name or the URL provided by your administrator.
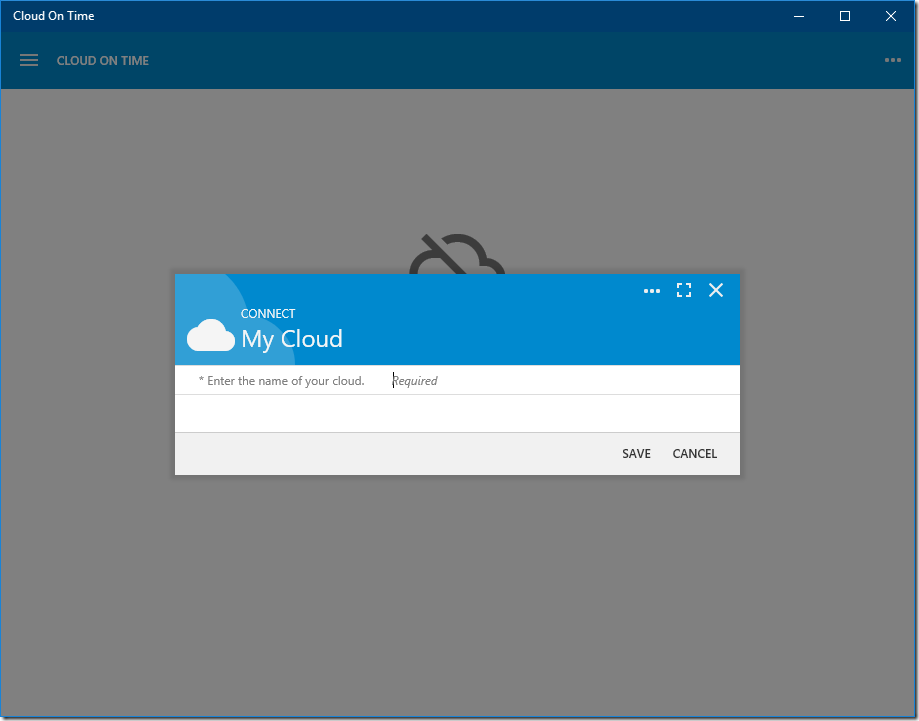
Tap “Save” to connect to the application in the cloud. The name, icon, and description of the compatible application will be displayed. If the information is correct, then tap OK.
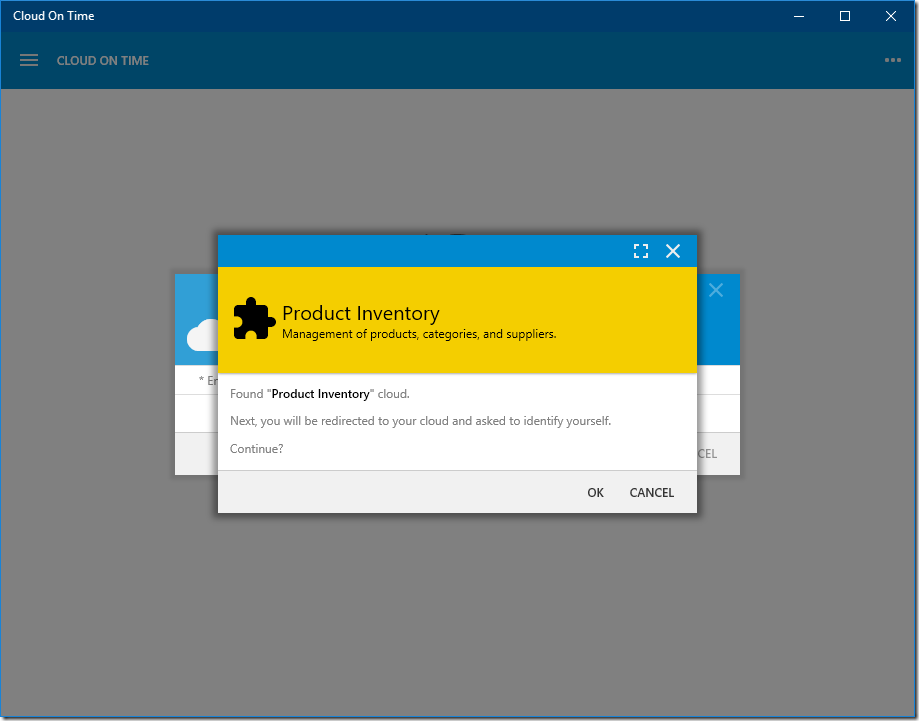
Cloud On Time app will redirect to the application in the cloud to confirm your identity. Enter the username and password for your account to authorize access on the device.
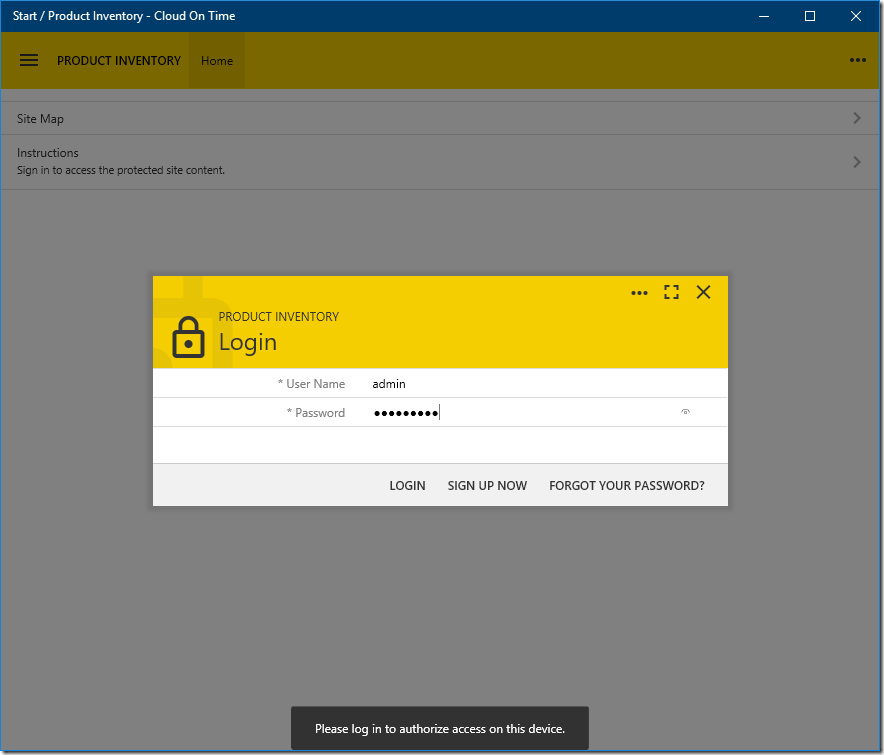
After receiving confirmation of user identity, Cloud On Time app will download the files of cloud application front-end and store them on the device. If the cloud application is configured to work in offline mode, then the data matched with the user identity is also downloaded.
The application is launched automatically after successful installation.
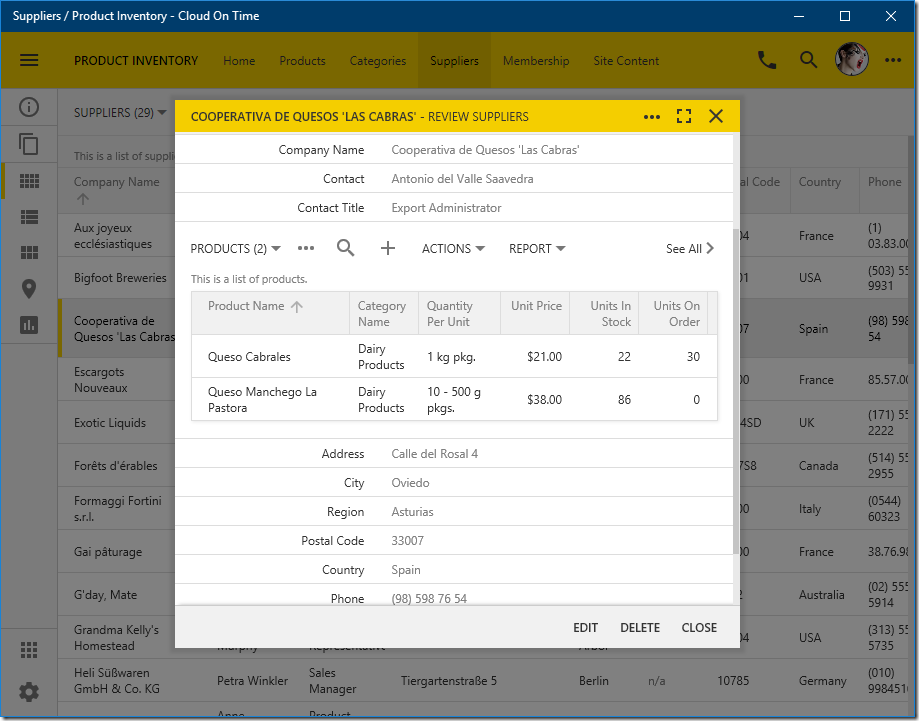
If you close the Code On Time app and start it again, then the installed cloud is automatically displayed with the same identity that was confirmed during the front-end installation process.
Cloud On Time app will also perform a brief verification of application files by comparing them with those reported by the application in the cloud. Any changes are automatically downloaded before the front-end is displayed.
File verification with the server is skipped if the app front-end is working with local data in offline mode. The process will be performed when the local data is synchronized and successfully committed to the cloud.
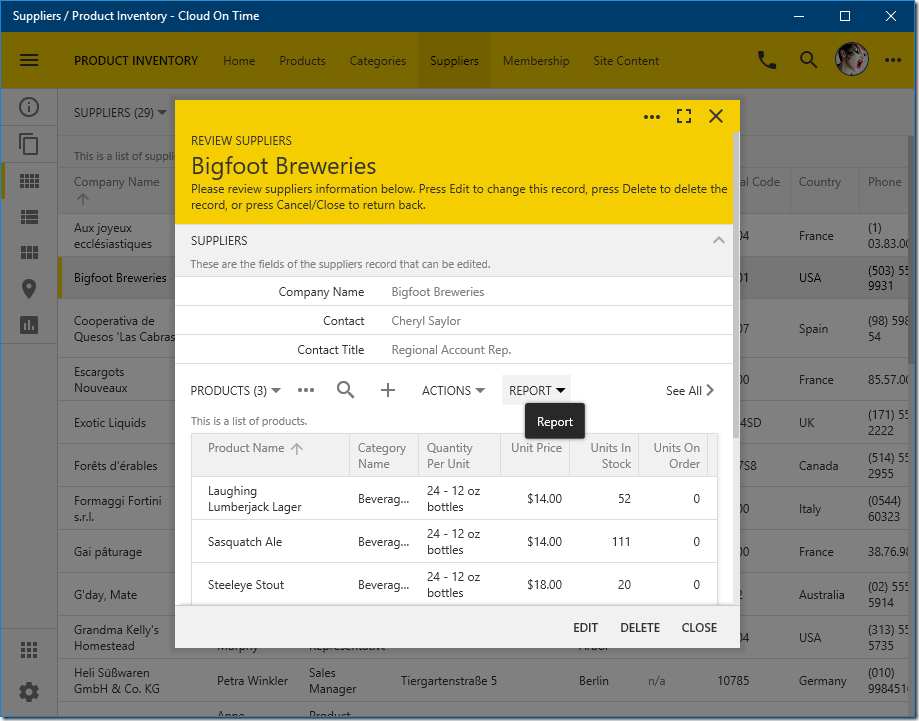
If you need more than one identity to work with the same app, then add another account by choosing “Add Account” option in the user menu.
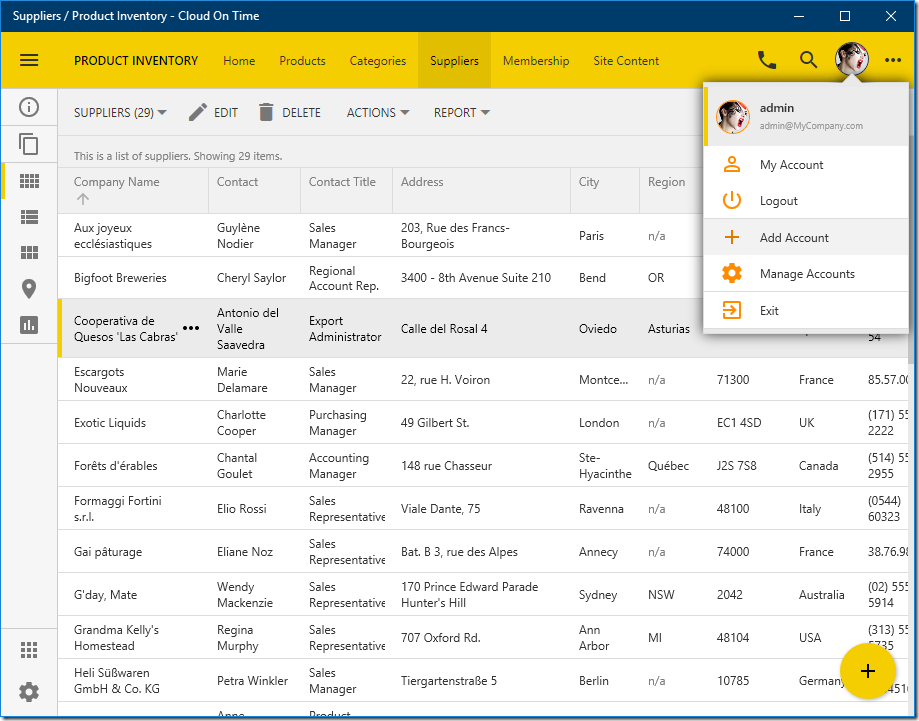
Confirm the new identity and the Cloud On Time app will install any required unique files on the device.
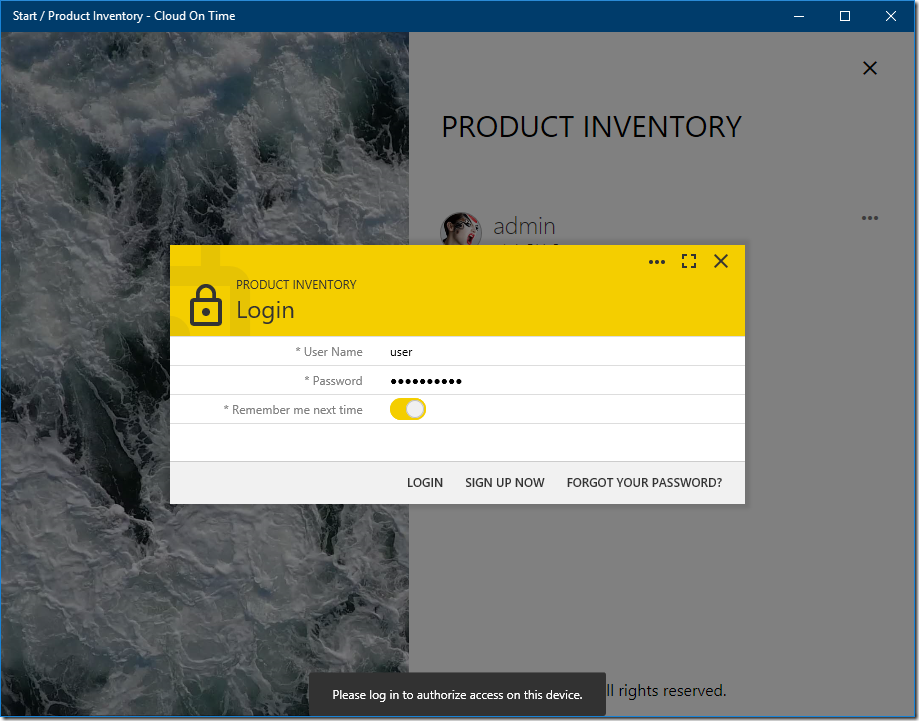
A local version of the front-end specific to the identity is launched.
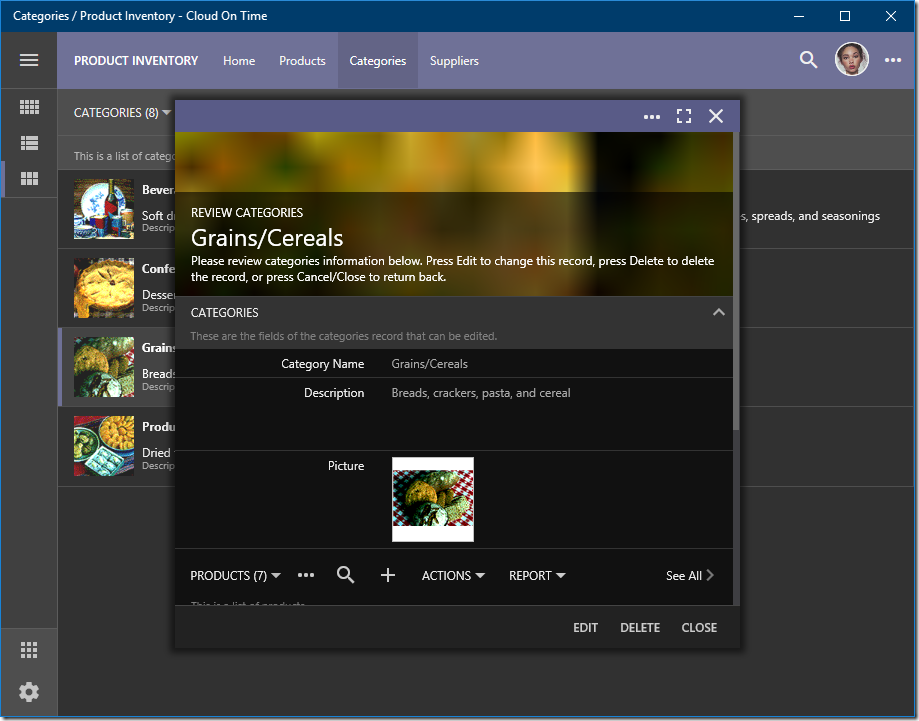
Use account manager to switch between identities.
If the Cloud On Time app is restarted, then the last used front-end with the last selected identity is launched automatically.
Choose “Exit” option in the user menu to exit the front-end and return to the home screen of Cloud On Time app.
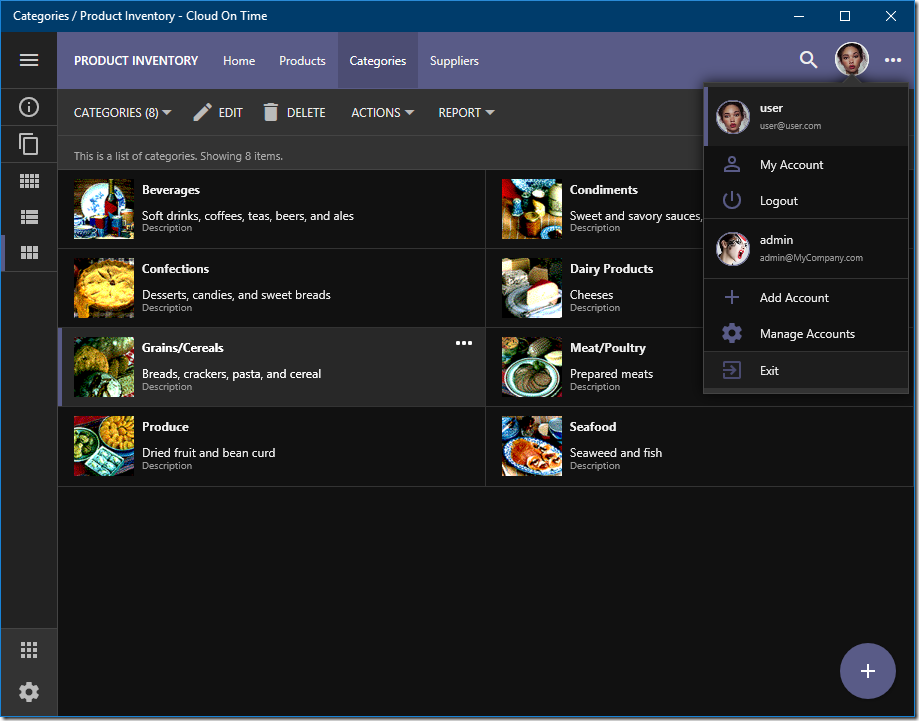
You will see a tile representing the application front-end on the home screen. Tap the tile to launch the front-end of the cloud application.
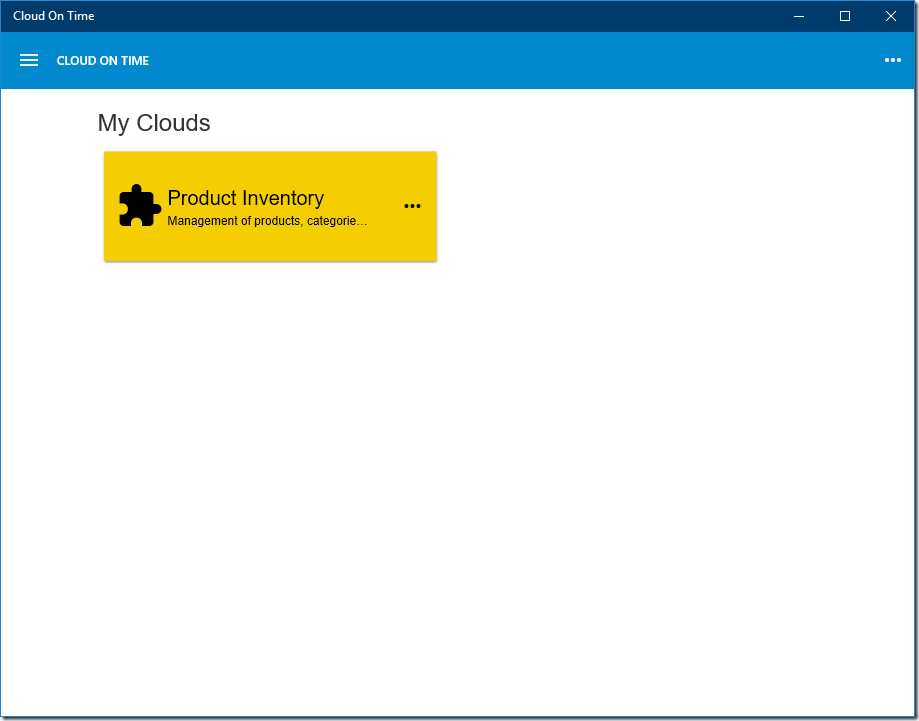
Use “more” button on the right side of the toolbar and press “Connect a Cloud” to connect another cloud to the home screen.
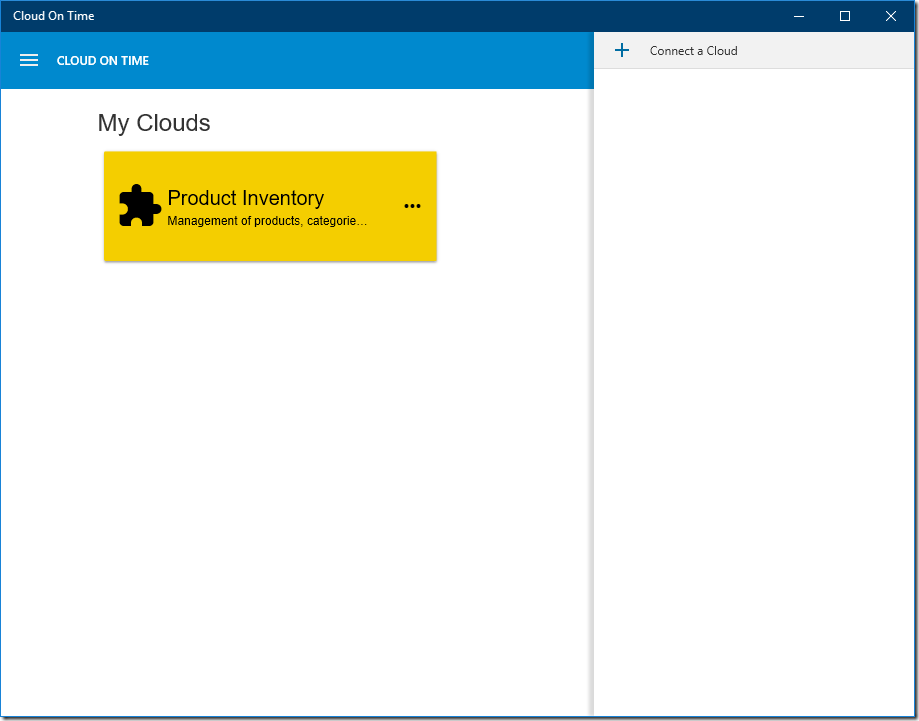
Enter the name or URL of the cloud application when prompted. Connect the app and proceed to confirm your identity.
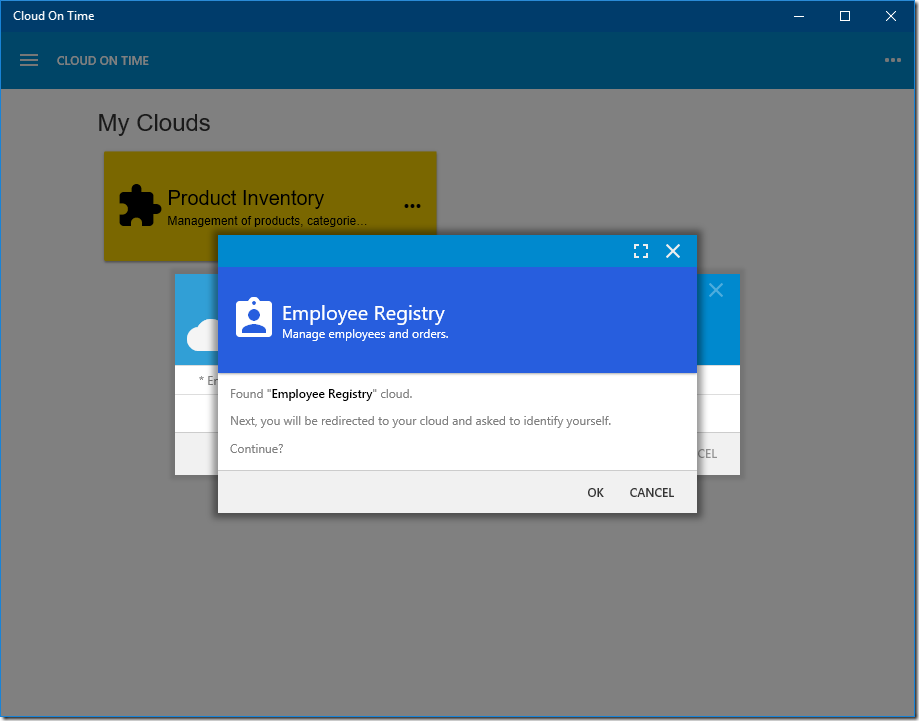
Cloud On Time app will display the front-end of the connected cloud application.
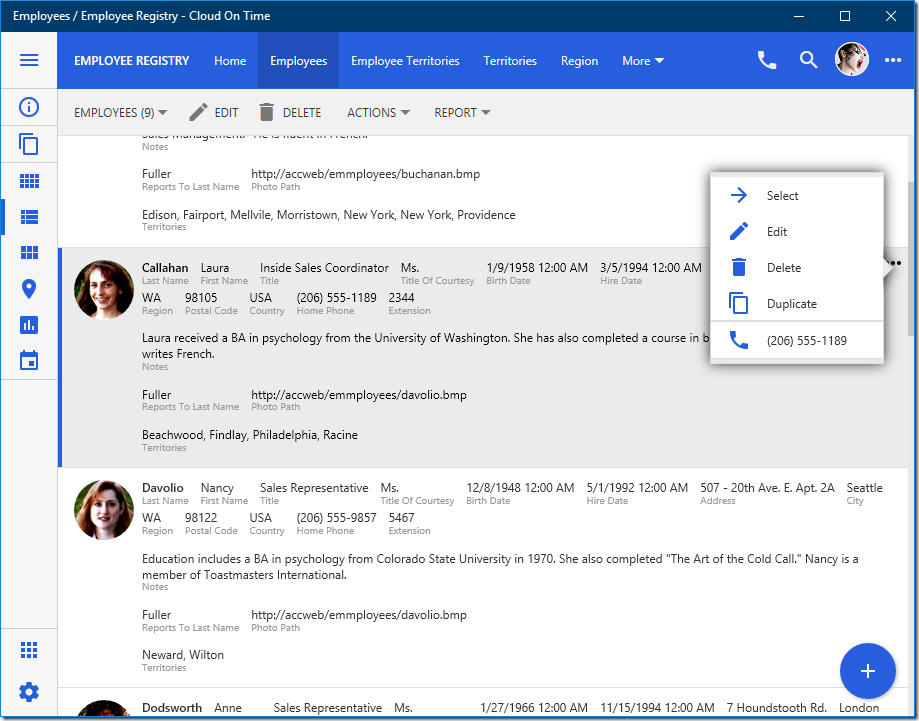
Use “Exit” command in the user menu to return to the home screen of Cloud On Time app.
Each tile on the home screen provides “more” button with options to run the front-end or to delete the connected app.
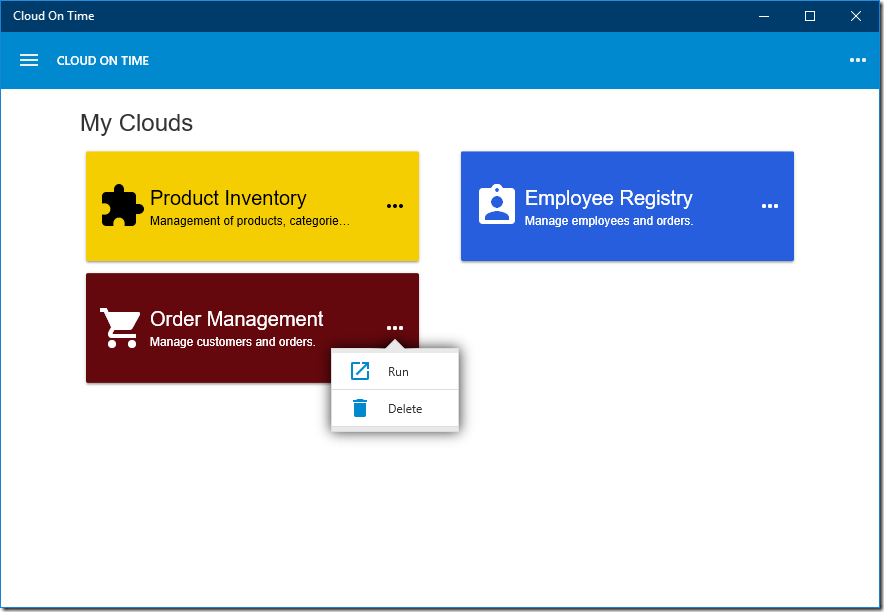
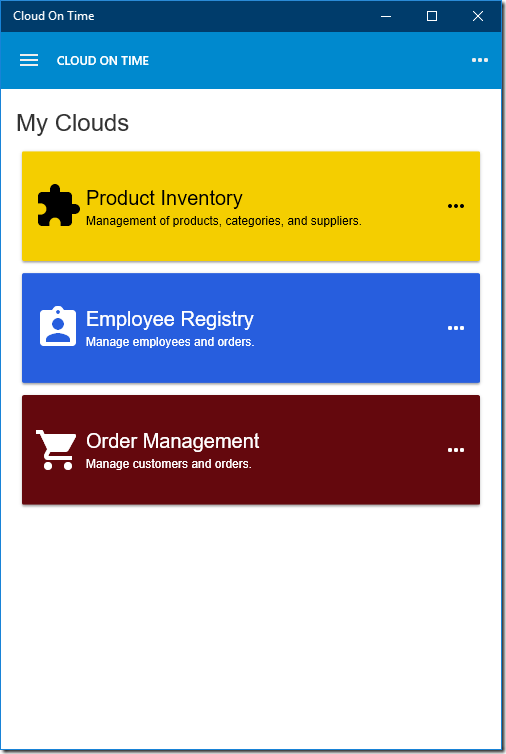
To prevent unauthorized access to the application, use Logout option in the user menu.
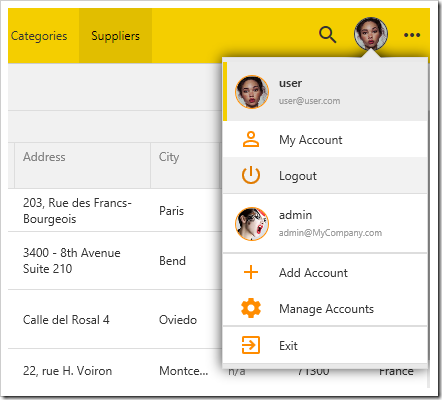
You will return to the home screen of Cloud On Time app. The tile of the cloud application will remain on the home screen.
An attempt to launch the “logged out” cloud application front-end will require confirmation of user identity in the cloud. When confirmed, the required files will be re-installed from the local cache on the device. The front-end will be launched with a minimal delay.
The cloud application can be configured by administrator to always confirm the user identity on the device when the front-end is started and to log the user out when the app is closed.