Code On Time applications support custom field editors. Use custom field editors to enhance the standard user interface of data fields in generated applications with custom presentation.
Background
Custom field editor is an ASP.NET User Control that has its code behind class declared with YourNamespace.Web.AquariumFieldEditor attribute. The attribute definition is generated as a part of the project code base.
Each generated application includes a standard custom field editor ~/Controls/RichEdtior.ascx. This user control hosts HtmlEditor component from Ajax Control Toolkit included with the generated project.
You can activate this field editor using one of the two methods.
- First method is to enter “RichEditor” in Editor property of a field that must support rich editing capability. You may also want to ensure that every instance of a field binding to a view has its HtmlEncoding attribute set to “false”. Field binding are data fields declared in each view of a data controller.
- The second method consists of setting the Text Mode property of a data field in a corresponding view to “Rich Text”. The client library will automatically handle configuring of Html Encoding and Editor properties at runtime.
Custom field editor can be implemented with the standard ASP.NET controls and requires a small amount of JavaScript code discussed below.
Custom Rich Editor Example
Let’s replace the standard HtmlEditor with UltimateEditor from Karamasoft to illustrate the basic steps required to integrate external components of the custom field editor.
Generate an ASP.NET 3.5 version of Web App Factory from your database. We will be using the Northwind sample for the purpose of this discussion. Designate a data field in one of the form views to have its Text Mode property set to “Rich Text” (/blog/2010/11/rich-text-editor.html). We will do so for Categories.Description field. Here is the form view editForm1 with Description field rendered as a standard Rich Text editor.
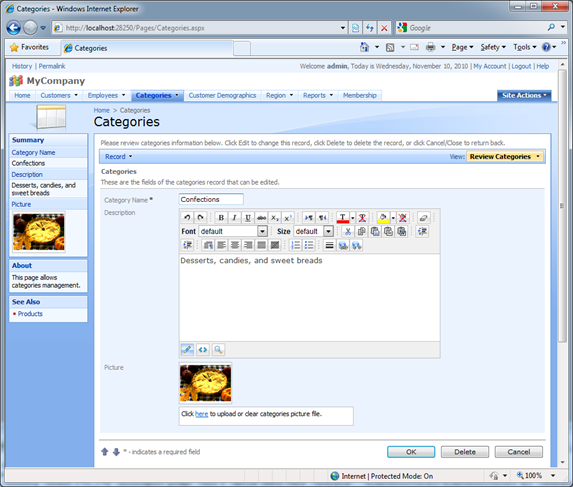
Download and install the Karamasoft Ultimiate Editor for ASP.NET 3.5. The installation program will save the files to the C:\inetpub folder on your computer. Consult installation documentation provided by the vendor and copy the following files to the generated project source code.
- Copy ephtmltopdf.dll, UltimateEditor.dll, and UltimateSpell.dll to the [Your Project Root]\WebApp\bin folder.
- Copy entire UltimateEditorInclude folder to the [Your Project Root]\WebApp folder.
- Copy entire UltimateSpellInclude folder to the [Your Project Root]\WebApp folder.
Open the project in Visual Studio 2008 and add the references to the DLLs copied in step 1 to your own project, include the folders copied in steps 2-3 into your project using the Solution Explorer.
The project WebApp of the generated solution will look as shown below:
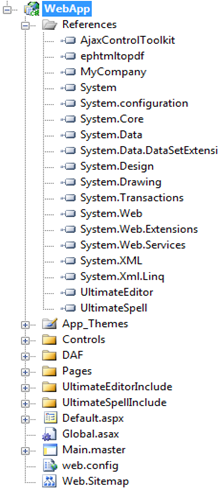
Open WebApp/Controls/RichEdit.ascx and the change the markup as shown in this C# sample.
<%@ Control Language="C#" AutoEventWireup="True" Inherits="Controls_RichEditor"
Codebehind="RichEditor.ascx.cs" %>
<%@ Register Assembly="UltimateEditor"
Namespace="Karamasoft.WebControls.UltimateEditor"
TagPrefix="kswc" %>
<kswc:UltimateEditor ID="UltimateEditor1" runat="server" SetFocus="False">
<UltimateSpell MisspelledWordStyle-Font-Bold="True"
MisspelledWordStyle-ForeColor="#FF0000"
GrammaticalErrorStyle-Font-Bold="True"
GrammaticalErrorStyle-ForeColor="#008000">
<SpellButton ButtonType="Button" Text="Spell Check"
CausesValidation="True"
ImageAlign="AbsMiddle"></SpellButton>
<GrammarButton ButtonType="Button" Text="Grammar Check"
CausesValidation="True"
ImageAlign="AbsMiddle"></GrammarButton>
</UltimateSpell>
</kswc:UltimateEditor>
Open the code-behind class and change it as shown in this example.
[MyCompany.Web.AquariumFieldEditor()]
public partial class Controls_RichEditor : System.Web.UI.UserControl
{
protected void Page_Load(object sender, EventArgs e)
{
if (!(IsPostBack))
Page.ClientScript.RegisterClientScriptBlock(GetType(), "ClientScripts", String.Format(@"
var initialValue = null;
function FieldEditor_GetValue(){{
if(typeof(UltimateEditors)!=='undefined')
return UltimateEditors['{0}'].GetEditorHTML();
else
return initialValue;
}}
function FieldEditor_SetValue(value) {{
if(typeof(UltimateEditors)!=='undefined')
UltimateEditors['{0}'].SetEditorHTML(value);
else {{
initialValue = value;
window.setTimeout('FieldEditor_SetValue(initialValue)', 100);
}}
}}
",
UltimateEditor1.ClientID), true);
}
}
The markup of the user control is quite obvious. Ultimate Editor is initialized with default property values. The most important one is probably the SetFocus property. We don’t want this control to take control over input focus from the client library of the generated application.
The source of the user control emits two JavaScript functions, FieldEditor_GetValue and FieldEditor_SetValue that are provided to allow integration with the client library of your application. The emitted functions will look as follows when the page hosting the user control is rendered in a browser:
var initialValue = null;
function FieldEditor_GetValue(){
if(typeof(UltimateEditors)!=='undefined')
return UltimateEditors['ctl03_UltimateEditor1'].GetEditorHTML();
else
return initialValue;
}
function FieldEditor_SetValue(value) {
if(typeof(UltimateEditors)!=='undefined')
UltimateEditors['ctl03_UltimateEditor1'].SetEditorHTML(value);
else {
initialValue = value;
window.setTimeout('FieldEditor_SetValue(initialValue)', 100);
}
}
The functions are using the Ultimate Editor API to interact with the client-side editor component of the vendor.
Note the use of window.setTimeout function that performs a delayed initialization of the vendor control. This technique may be needed if the the vendor control is dynamically loading its own scripts and is not ready for use upon initial loading of the editor in the browser window. Such situation may occur upon first activation of the editor – subsequent activations do not require a delay.
The last step is ensure that enough of the page real estate is available for Ultimate Editor at runtime. Add a new CSS file to the WebAp/App_Themes folder of your solution.
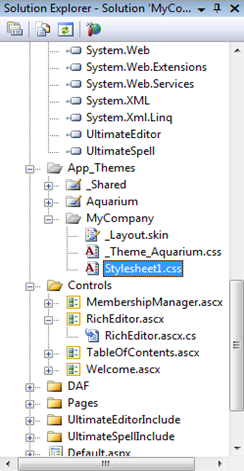
Enter the following CSS rule:
iframe.FieldEditor
{
width: 695px !important;
height: 379px !important;
}
Run the WebApp project to see the new version of Rich Editor control in action.
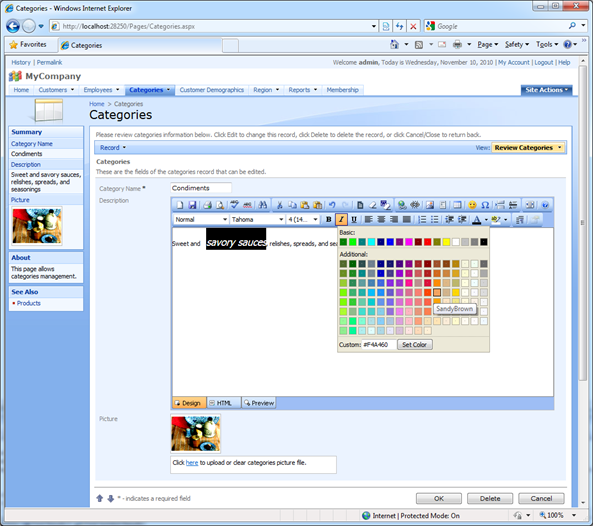
Subsequent code generation of your Web App Factory project will retain all project modifications. The code generator will use the latest version of the project file to list the same folders and references that were included in the project for the purpose of this exercise.