Let’s create a page that will contain a custom user control and a data view. The user control will contain several buttons that will programmatically change the filter on the data view.
Adding the Page
Start the Project Designer. On the Project Explorer toolbar, click on the New Page icon.
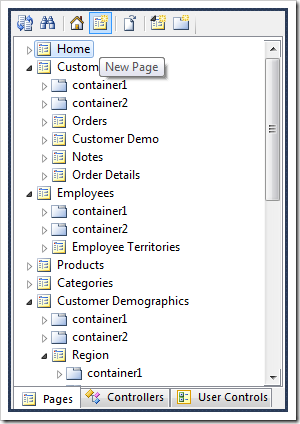
Assign a name to the page:
Property | Value |
Name | Custom Filter Panel |
Press OK to save. Drop the Custom Filter Panel page node on the right side of Home page node to place it second in the menu.
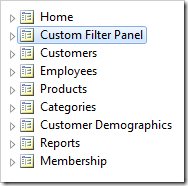
Right-click on Custom Filter Panel node, and press New Container.
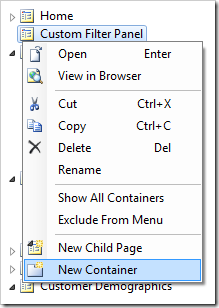
Keep the default configuration and press OK to save the container. Right-click on Custom Filter Panel / c101 container node, and press New Control.
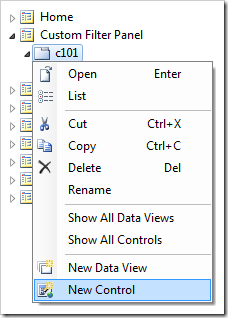
Next to the User Control lookup, click on the New User Control icon.
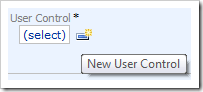
Assign the user control a name:
Property | Value |
Name | FilterPanel |
Press OK to create the new user control. Press OK to add the control to the page.
Implementing the User Control
On the Project Designer toolbar, press Browse to generate the application and user control file.
When complete, right-click on Custom Filter Panel / c101 / control1 – FilterPanel node and press Edit in Visual Studio.
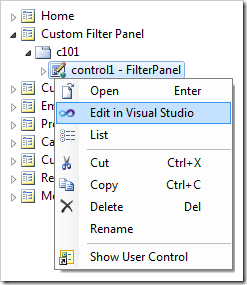
The user control file will be opened in Visual Studio. Replace the code base after the <%@ Control %> element with the following:
<asp:UpdatePanel ID="UpdatePanel1" runat="server">
<ContentTemplate>
<div style="margin-bottom:4px">
<h2 style="margin-bottom:4px; margin-top:6px;">Select a filter:</h2>
<asp:Button ID="Button1" runat="server" OnClick="Button1_Click" Text="Shipped" />
<asp:Button ID="Button2" runat="server" OnClick="Button2_Click" Text="Not Shipped" />
<asp:Button ID="Button3" runat="server" OnClick="Button3_Click" Text="High Freight" />
<asp:Button ID="Button4" runat="server" OnClick="Button4_Click" Text="Reset" />
</div>
</ContentTemplate>
</asp:UpdatePanel>
<div id="OrderList" runat="server">
</div>
<aquarium:DataViewExtender ID="dve1" runat="server" TargetControlID="OrderList"
Controller="Orders" />
The code above will add four buttons and an Orders data view. Right-click and press View Code.
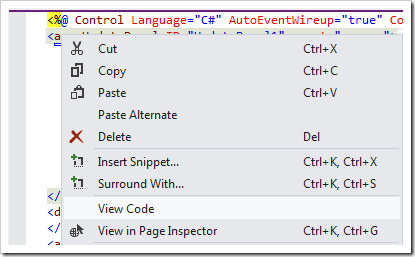
Replace the existing code base with the following:
C#:
using System;
using System.Collections.Generic;
using System.Linq;
using System.Text.RegularExpressions;
using System.Web;
using System.Web.UI;
using System.Web.UI.WebControls;
using MyCompany.Web;
using MyCompany.Data;
public partial class Controls_FilterPanel : System.Web.UI.UserControl
{
// assign filter when page loads
protected void Page_Load(object sender, EventArgs e)
{
if (!IsPostBack)
dve1.AssignStartupFilter(new FieldFilter[] {
new FieldFilter(
"ShippedDate",
RowFilterOperation.DoesNotInclude,
null) });
}
// filter ShippedDate to not null
protected void Button1_Click(object sender, EventArgs e)
{
dve1.AssignFilter(new FieldFilter[] {
new FieldFilter(
"ShippedDate",
RowFilterOperation.DoesNotInclude,
null),
new FieldFilter(
"Freight",
RowFilterOperation.None) });
}
// filter ShippedDate to null
protected void Button2_Click(object sender, EventArgs e)
{
dve1.AssignFilter(new FieldFilter[] {
new FieldFilter(
"ShippedDate",
RowFilterOperation.Equal,
null),
new FieldFilter(
"Freight",
RowFilterOperation.None) });
}
// filter Freight to >30
protected void Button3_Click(object sender, EventArgs e)
{
dve1.AssignFilter(new FieldFilter[] {
new FieldFilter(
"Freight",
RowFilterOperation.GreaterThan,
30),
new FieldFilter(
"ShippedDate",
RowFilterOperation.None)});
}
// reset filter when Reset button clicked
protected void Button4_Click(object sender, EventArgs e)
{
dve1.AssignFilter(new FieldFilter[] {
new FieldFilter(
"ShippedDate",
RowFilterOperation.None),
new FieldFilter(
"Freight",
RowFilterOperation.None) });
}
}
Visual Basic:
Imports System
Imports System.Collections.Generic
Imports System.Linq
Imports System.Text.RegularExpressions
Imports System.Web
Imports System.Web.UI
Imports System.Web.UI.WebControls
Imports MyCompany.Web
Imports MyCompany.Data
Partial Public Class Controls_FilterPanel
Inherits Global.System.Web.UI.UserControl
' assign filter when page loads
Protected Sub Page_Load(ByVal sender As Object, ByVal e As EventArgs) Handles Me.Load
If Not IsPostBack Then
dve1.AssignStartupFilter(New FieldFilter() {
New FieldFilter(
"ShippedDate",
RowFilterOperation.Equal,
Nothing)})
End If
End Sub
' filter ShippedDate to not null
Protected Sub Button1_Click(sender As Object, e As EventArgs)
dve1.AssignFilter(New FieldFilter() {
New FieldFilter(
"ShippedDate",
RowFilterOperation.NotEqual,
Nothing),
New FieldFilter(
"Freight",
RowFilterOperation.None)})
End Sub
' filter ShippedDate to null
Protected Sub Button2_Click(sender As Object, e As EventArgs)
dve1.AssignFilter(New FieldFilter() {
New FieldFilter(
"ShippedDate",
RowFilterOperation.Equal,
Nothing),
New FieldFilter(
"Freight",
RowFilterOperation.None)})
End Sub
' filter Freight to >30
Protected Sub Button3_Click(sender As Object, e As EventArgs)
dve1.AssignFilter(New FieldFilter() {
New FieldFilter(
"ShippedDate",
RowFilterOperation.None),
New FieldFilter(
"Freight",
RowFilterOperation.GreaterThan,
30)})
End Sub
' reset filter when Reset button clicked
Protected Sub Button4_Click(sender As Object, e As EventArgs)
dve1.AssignFilter(New FieldFilter() {
New FieldFilter(
"ShippedDate",
RowFilterOperation.None),
New FieldFilter(
"Freight",
RowFilterOperation.None)})
End Sub
End Class
The code will assign filters when any of the buttons are pressed. Save the file.
Viewing the Results
Switch to the open web application and refresh the page. Navigate to Custom Filter Panel page. The page will contain four buttons and an Orders data view. The data view will be filtered to show only rows that have a value in Shipped Date field.
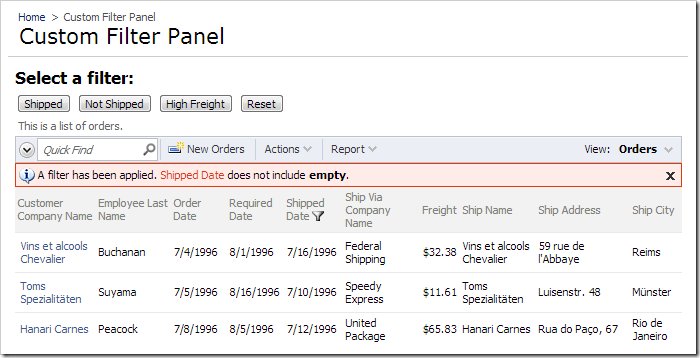
Click on one of the first three buttons. The filter will change accordingly.
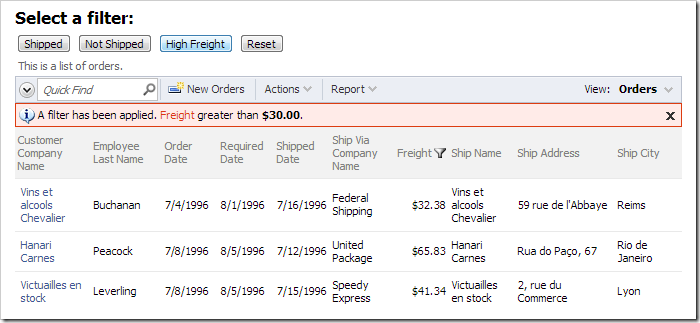
Clicking on the Reset button will clear all filters.
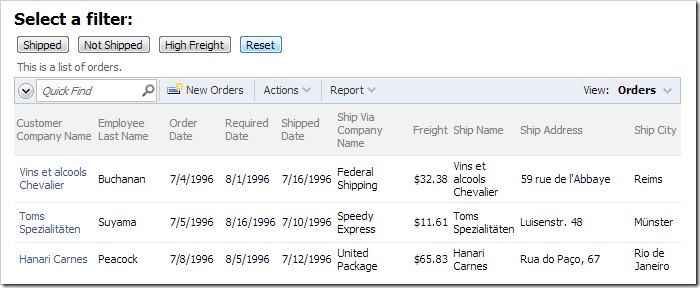