Code On Time web applications support out-of-the-box reporting capabilities that require zero programming.
Standard Report Actions
All data views offer four standard actions that yield a different output.
- Action “ReportAsPdf” will render the data presented to end users as a Adobe PDF document. The shorthand action “Report” will produce the same result. This type of report requires a compatible software installed on the client computer to view and print the report output. You can download free Adobe Acrobat Reader at at http://get.adobe.com/reader.
- Action “ReportAsImage” creates a TIFF image file, which requires a compatible software installed on the client. TIFF format supports multiple pages and is a perfect alternative to PDF. The quality of output in PDF and TIFF formats is equivalent.
- Action “ReportAsWord” renders a report as Microsoft Word document with a high-quality output. In some instances the output is less precise then the output produced by the action “ReportAsImage” and “ReportAsPdf”.
- Action “ReportAsExcel” produces a Microsoft Excel spreadsheet that offer a lesser quality output due to rendering restriction of the popular spreadsheet software.
If a user requests a “Report...” action then a report is rendered on the server with the help of Microsoft Report Viewer. The output is streamed to the client browser. Typically a prompt is displayed before a compatible installed software viewer will be activated. Users also have an option to save the output locally. If a viewer is not installed on the client computer then the prompt to save the file is the only option.
Code On Time web applications execute various server calls off-band to provide smooth Web 2.0 user experience. Modern web browsers make sure to prevent all sorts of popups initiated by the scripts embedded in the web pages. The client library makes use of correct techniques to process reports on the server without causing automatic activation of popup blockers.
Custom Report Action Handlers
Code On Time web applications created with version 6.0.0.19 or higher allow developers to perform custom processing of reporting actions in business rules. There are several reasons that may require an execution of custom code that must precede or override the standard report rendering logic:
- The data must be “prepared” before a report is rendered.
- The report request must be logged.
- An external report rendering engine is available. The custom code will redirect the report request to such an engine.
- A special report preview page needs to be displayed.
- A custom report building code must execute. The custom code replaces the standard report rendering logic.
Create a business rule for your data controller and implement methods to handle the corresponding “Report...” actions when you need to override the standard report processing.
Example
Generate a Northwind sample using Code On Time web application generator.
Select the project name on the start page of the code generator and click Design.
Select Controllers tab in the Project Explorer and enter CustomerBusinessRules in the Handler property of the Customers data controllers. Click OK to save the changes.
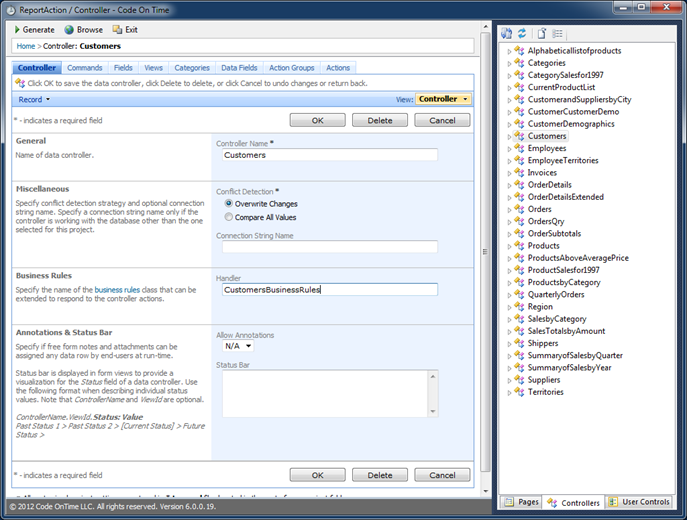
Expand Actions node of the Customers data controller in Project Explorer, right-click Actions and create a new action group with the Scope of Action Column.
Add a new action in the newly created action group, set its Command Name to Report, and Command Argument to _blank.
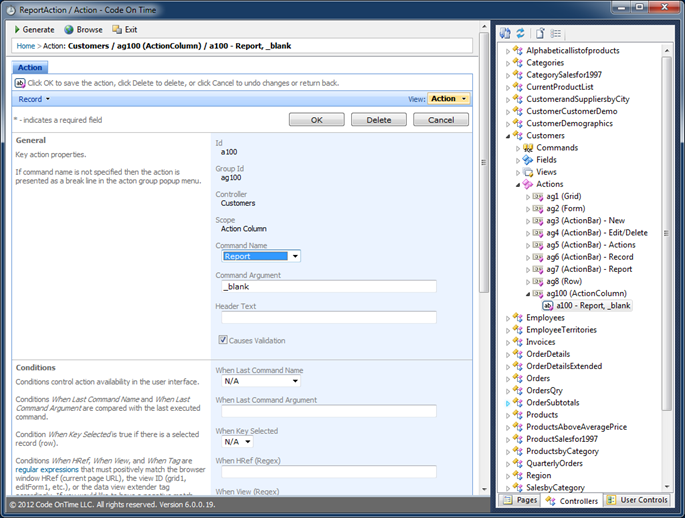
Here is the sub-tree of the Customers data controller with the Report action selected.
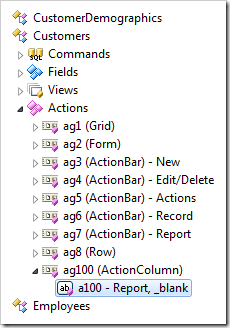
Click Exit on the Designer tool bar and generate your application.
Select the project name on the start page of the web application generator and choose Develop. Visual Studio or Visual Web Developer will start.
Locate the file ~/App_Code/Rules/CustomerBusinessRules.cs(vb) and enter the following code.
C#:
using System;
using System.Data;
using System.Collections.Generic;
using System.Linq;
using MyCompany.Data;
using System.IO;
namespace MyCompany.Rules
{
public partial class CustomersBusinessRules : MyCompany.Data.BusinessRules
{
[ControllerAction("Customers", "Report", "_blank")]
public void RedirectToCustomReport(string customerId, string companyName)
{
// Redirect user to another URL
Result.NavigateUrl = String.Format(
"~/Pages/Customers.aspx?CustomerID={0}&_controller=Customers" +
"&_commandName=Select&_commandArgument=editForm1",
customerId);
}
[ControllerAction("Customers", "ReportAsImage", "")]
public void GenerateCustomReport(string customerId, string companyName)
{
PreventDefault();
// return the same image in response to all "Report..." commands
Context.Response.Clear();
Context.Response.ContentType = "image/jpeg";
Context.Response.AddHeader("Content-Disposition",
String.Format("attachment;filename={0}.jpg", customerId));
byte[] reportData =
File.ReadAllBytes(@"C:\Users\Public\Pictures\Sample Pictures\Koala.jpg");
Context.Response.AddHeader("Content-Length", reportData.Length.ToString());
Context.Response.OutputStream.Write(reportData, 0, reportData.Length);
}
}
}
Visual Basic:
Imports MyCompany.Data
Imports System
Imports System.Collections.Generic
Imports System.Data
Imports System.Linq
Imports System.IO
Namespace MyCompany.Rules
Partial Public Class Customers
Inherits MyCompany.Data.BusinessRules
<ControllerAction("Customers", "Report", "_blank")> _
Sub RedirectToCustomReport(ByVal customerId As String, ByVal companyName As String)
' Redirect user to another URL
Result.NavigateUrl = String.Format( _
"~/Pages/Customers.aspx?CustomerID={0}&_controller=Customers" + _
"&_commandName=Select&_commandArgument=editForm1",
customerId)
End Sub
<ControllerAction("Customers", "ReportAsImage", "")> _
Sub GenerateCustomReport(ByVal customerId As String, ByVal companyName As String)
PreventDefault()
'return the same image in response to all "Report..." commands
Context.Response.Clear()
Context.Response.ContentType = "image/jpeg"
Context.Response.AddHeader("Content-Disposition", _
String.Format("attachment;filename={0}.jpg", customerId))
Dim reportData As Byte() = _
File.ReadAllBytes("C:\Users\Public\Pictures\Sample Pictures\Koala.jpg")
Context.Response.AddHeader("Content-Length", reportData.Length.ToString())
Context.Response.OutputStream.Write(reportData, 0, reportData.Length)
End Sub
End Class
End Namespace
The first method RedirectToCustomReport will use the ID of the selected customer to compose a URL relative to the application. The URL will open in a new browser window and will force the application to select a customer with the specified ID.
The new window will open if your have entered the command argument as “_blank” when defining the action in Project Designer. If action command argument has been left blank then the new URL will replace the page in the web browser. Users will have an option to return to the previous page using the browser’s Back button.
The screen shot of the Customers page prior to execution of our Report action is shown next.
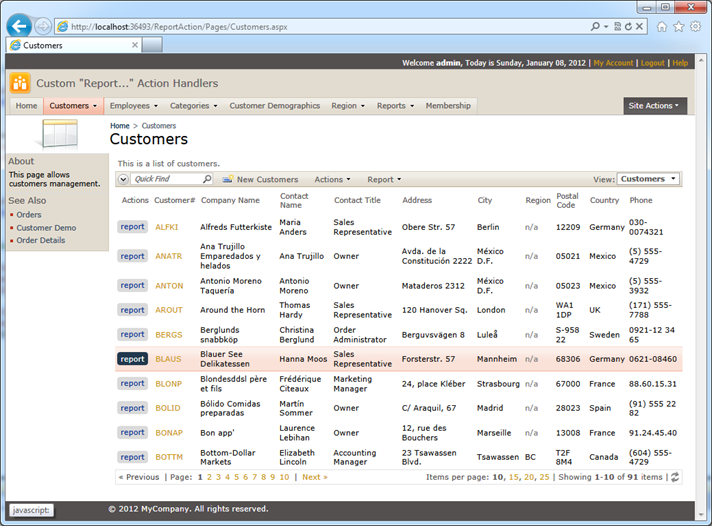
If you click on the Report button then a new browser window will open. You can see the address bar reflecting the ID of the selected customer.
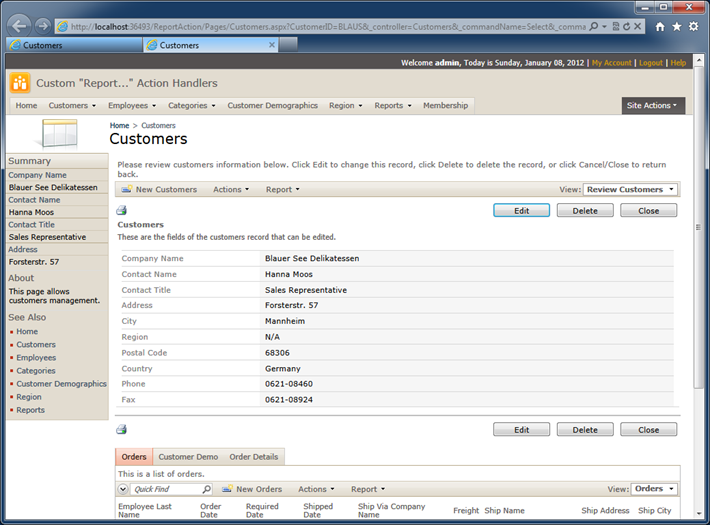
Our web application is using its own capabilities to present the data with the help of data controller URL parameters. You can redirect the report action to a generic web request handler or to a web-enabled report server such as Crystal Reports or Microsoft SQL Server Reporting Services.
The second method GenerateCustomReport takes control over the report rendering completely.
First, the method cancels out the default reporting logic at the very beginning by calling PreventDefault.
Next it reads the file C:\Users\Public\Pictures\Sample Pictures\Koala.jpg and streams it out. In a real-world application you can produce any sort of output using a custom code.
The method uses the customer ID to assign the file name to the output.
If you are reproducing this sample on a Windows 7 computer then there is not need to change the code . Otherwise change the path to the image file accordingly.
Run your web app, navigate to Customers page and select a customer.
Choose Report | Multipage Image on the action bar above the list of customers.
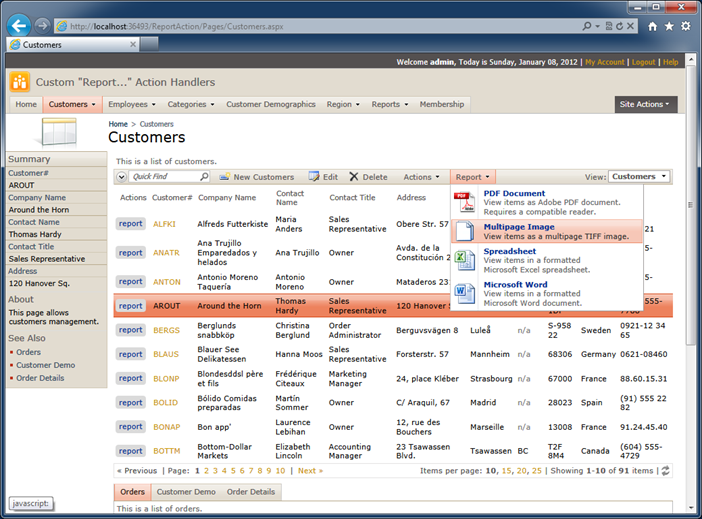
The custom method will execute and you will see the following prompt to download the file AROUT.jpg if you browsing with IE 9.
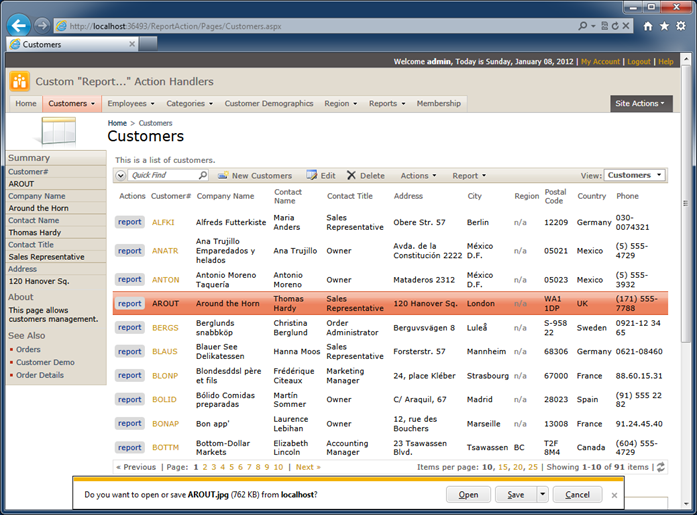
Click Open and the default image viewer will start.
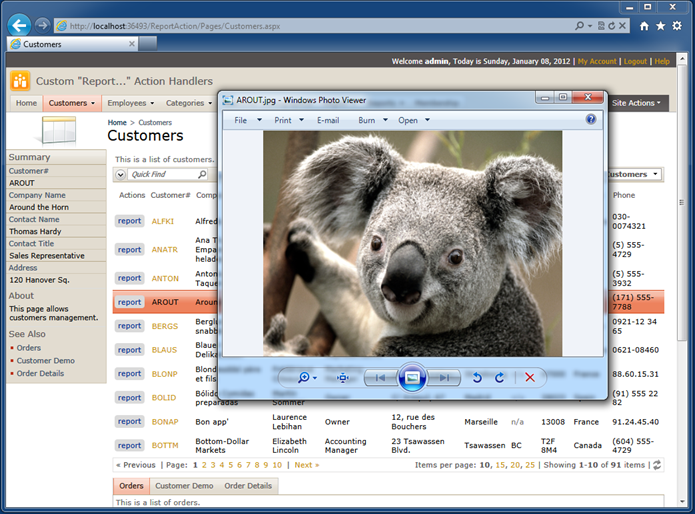
If you select any other customer and choose the same action bar option then exactly the same image will be downloaded but the file name will reflect the actual primary key of the selected customer.
In a real-world applications you will likely stream other formats of output such as PDF or custom Microsoft Office documents using 3rd party reporting software.