Conditional visibility on data fields and categories allows hiding or showing page elements based on field values. View templates give the ability to define custom presentations. When a custom template is defined for the view, the client library is not able to determine which page elements should be displayed or hidden based on the conditional visibility expressions. As such, the creator of view templates must mark up the template in order to bind these expressions with the correct elements.
When the template has been correctly defined, data fields, categories, and even custom page elements will be able to displayed or hidden depending on field values. For example, a large label is displayed in the sample below when the order has been shipped past the required date.
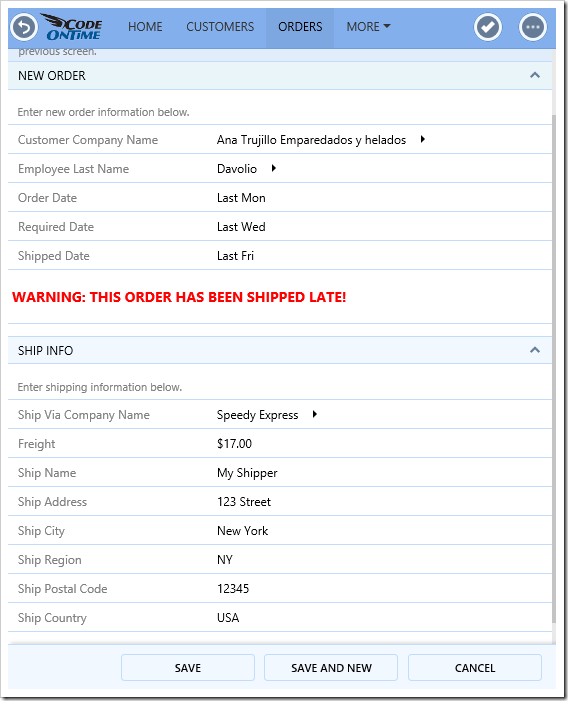
Let’s use the create form for Orders in the Northwind sample database.
Arranging Data Fields into Categories
First, let’s rearrange the data fields into multiple categories, in order to control visibility of each category, instead of each individual data field. The user will only be able to enter shipping information if a shipped date is assigned.
Start the Project Designer. In the Project Explorer, switch to the Controllers tab. Right-click on “Orders / createForm1” view node, and press New Category.
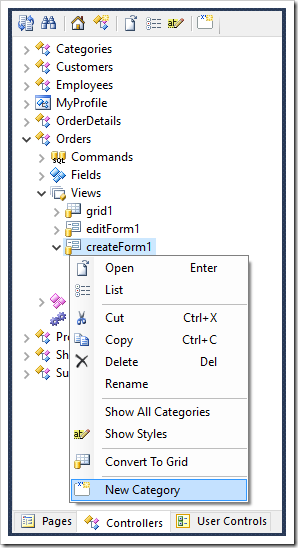
Define the following settings:
Property | Value |
Header Text | Ship Info |
Visible When | $row.ShippedDate != null
|
Press OK to save the category. Next, drag data fields ShipVia, Freight, ShipName, ShipAddress, ShipCity, ShipRegion, ShipPostalCode, and ShipCountry into the new category.
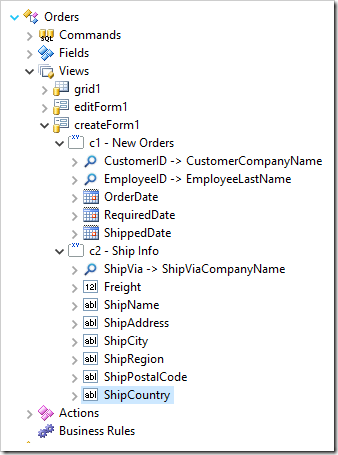
Adding Data Field Visibility
Users should not be able to set the shipped date until the order date has been set. Let’s add a data field conditional visibility expression to ShippedDate data field.
Double-click on “Orders / Views / createForm1 / c1 – New Orders / ShippedDate” data field node.
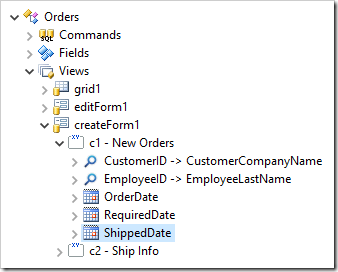
Make the following change:
Property |
Value |
Visible When |
$row.OrderDate != null
|
Press OK to save the data field.
Adding the View Template
Let’s add a custom view template for editForm1 of Orders controller.
On the toolbar, press Develop to open the project in Visual Studio. In the Solution Explorer, right-click on the “WebSite” node and press “Add / New Folder”.
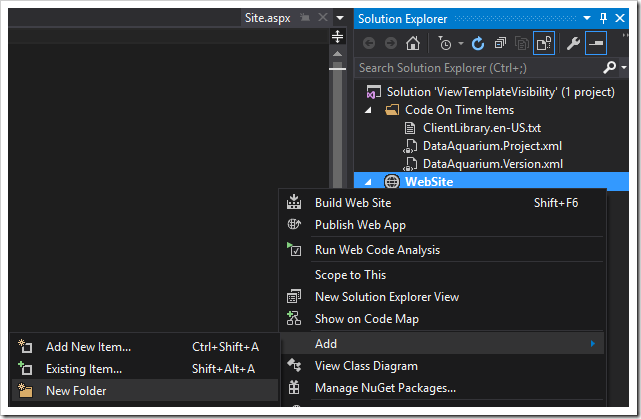
Give this new folder the name “Views”. Next, right-click on the folder and press “Add / HTML Page”.
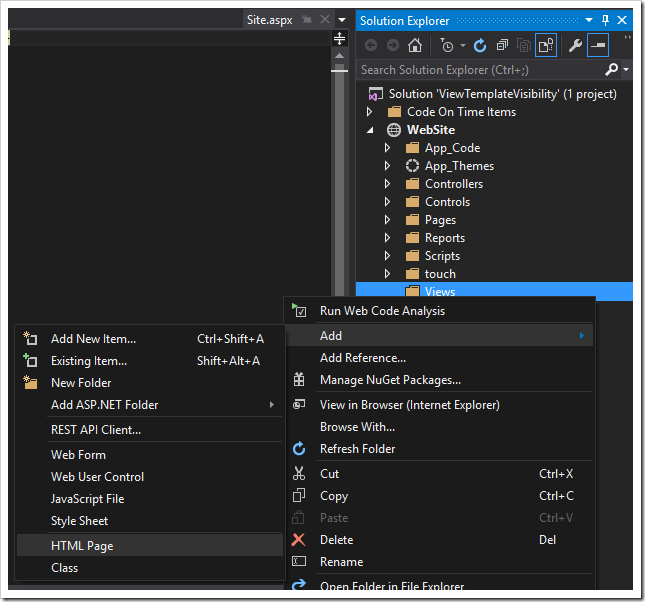
Give this page the name “Orders.createForm1.html”. Replace the contents of the file with the following:
<div data-container="collapsible" data-header-text="New Order">
<div data-container="row">
<div data-control="description">Enter new order information below.</div>
</div>
<div data-container="row">
<div data-control="label" data-field="CustomerID">CustomerID</div>
<div data-control="field" data-field="CustomerID">CustomerID</div>
</div>
<div data-container="row">
<div data-control="label" data-field="EmployeeID">EmployeeID</div>
<div data-control="field" data-field="EmployeeID">EmployeeID</div>
</div>
<div data-container="row">
<div data-control="label" data-field="OrderDate">OrderDate</div>
<div data-control="field" data-field="OrderDate">OrderDate</div>
</div>
<div data-container="row">
<div data-control="label" data-field="RequiredDate">RequiredDate</div>
<div data-control="field" data-field="RequiredDate">RequiredDate</div>
</div>
<div data-container="row" data-visibility="f:ShippedDate">
<div data-control="label" data-field="ShippedDate">ShippedDate</div>
<div data-control="field" data-field="ShippedDate">ShippedDate</div>
</div>
<div data-container="row" data-visible-when="$row.RequiredDate < $row.ShippedDate">
<h3 style="color:red">WARNING: THIS ORDER HAS BEEN SHIPPED LATE</h3>
</div>
</div>
<div data-container="collapsible" data-header-text="Ship Info" data-visibility="c:c2">
<div data-container="row">
<div data-control="description">Enter shipping information below.</div>
</div>
<div data-container="row">
<div data-control="label" data-field="ShipVia">ShipVia</div>
<div data-control="field" data-field="ShipVia">ShipVia</div>
</div>
<div data-container="row">
<div data-control="label" data-field="Freight">Freight</div>
<div data-control="field" data-field="Freight">Freight</div>
</div>
<div data-container="row">
<div data-control="label" data-field="ShipName">ShipName</div>
<div data-control="field" data-field="ShipName">ShipName</div>
</div>
<div data-container="row">
<div data-control="label" data-field="ShipAddress">ShipAddress</div>
<div data-control="field" data-field="ShipAddress">ShipAddress</div>
</div>
<div data-container="row">
<div data-control="label" data-field="ShipCity">ShipCity</div>
<div data-control="field" data-field="ShipCity">ShipCity</div>
</div>
<div data-container="row">
<div data-control="label" data-field="ShipRegion">ShipRegion</div>
<div data-control="field" data-field="ShipRegion">ShipRegion</div>
</div>
<div data-container="row">
<div data-control="label" data-field="ShipPostalCode">ShipPostalCode</div>
<div data-control="field" data-field="ShipPostalCode">ShipPostalCode</div>
</div>
<div data-container="row">
<div data-control="label" data-field="ShipCountry">ShipCountry</div>
<div data-control="field" data-field="ShipCountry">ShipCountry</div>
</div>
</div>
Notice that there are three highlighted pieces in the sample above.
The yellow highlight shows how to apply data field-level visibility to an element by using the attribute “data-visibility”, and setting the value to “f:” followed by the name of the field. This will inherit the visibility from the field “ShippedDate”.
The green highlight shows how to apply category-level visibility to an element. Use the attribute “data-visibility”, and set the value to “c:” followed by the category ID. The example will inherit visibility from the category “c2”.
The orange highlight shows how to use custom JavaScript expressions to set visibility. Use the attribute “data-visible-when”, and set the value to your JavaScript visibility expression.
Switch back to the browser, navigate to the Orders page, and create a new order. Notice that the OrderDate data field, custom header, and shipping category are hidden.
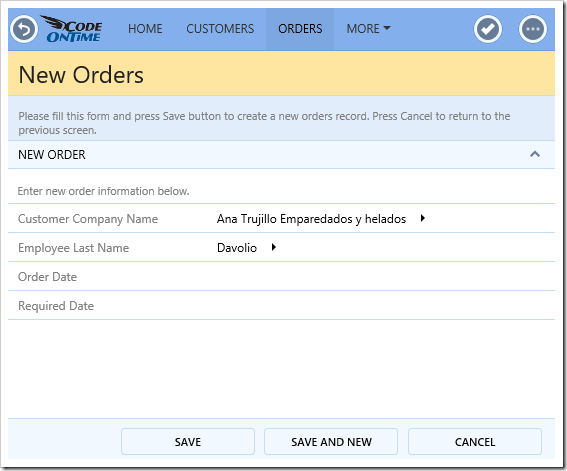
Enter a value for Order Date. Notice that the Shipped Date data field will appear.
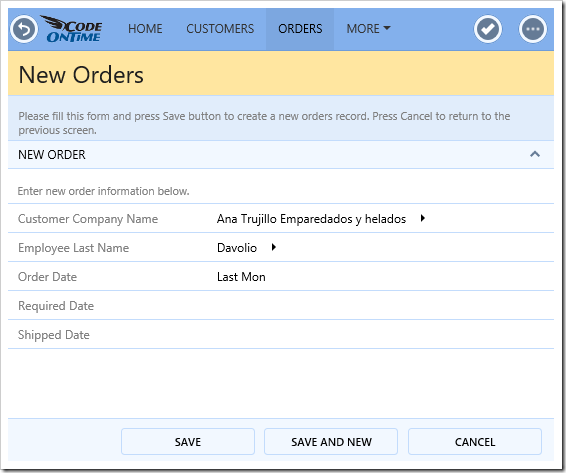
Enter a value for Shipped Date. The Ship Info category will appear.
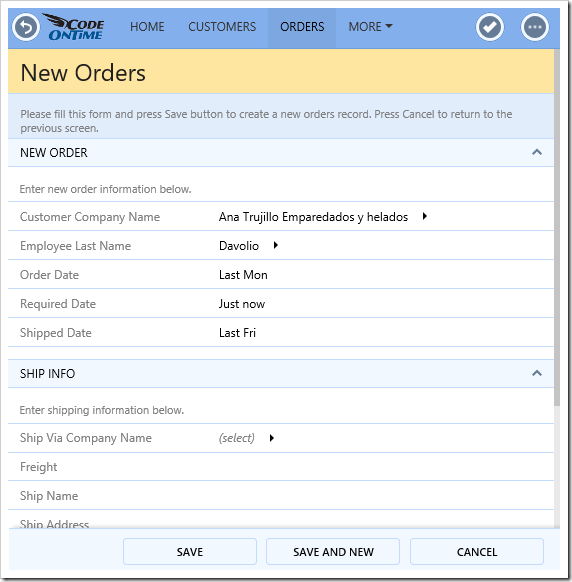
If the Shipped Date is after the Required Date, the warning text will appear.
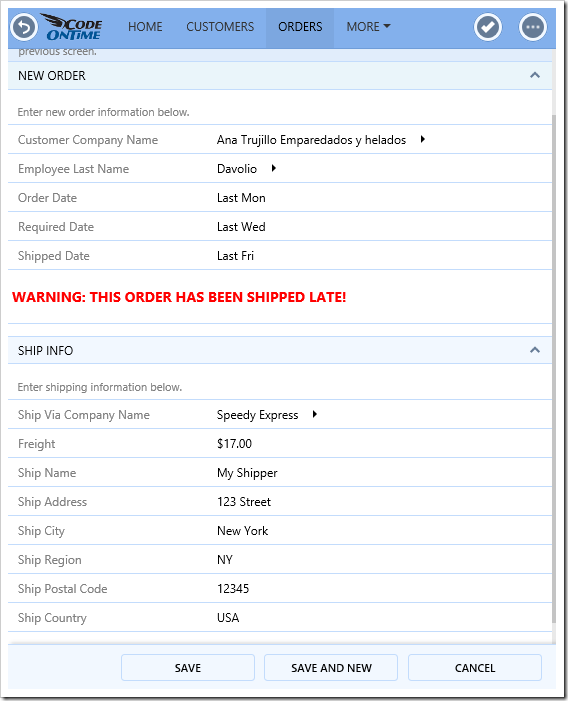