Client library automatically assigns a calendar behavior to all date-time input elements. This is the example of the calendar displayed next to input elements of Order Date, Required Date, and Shipped Date fields in the Orders form of the Northwind sample.
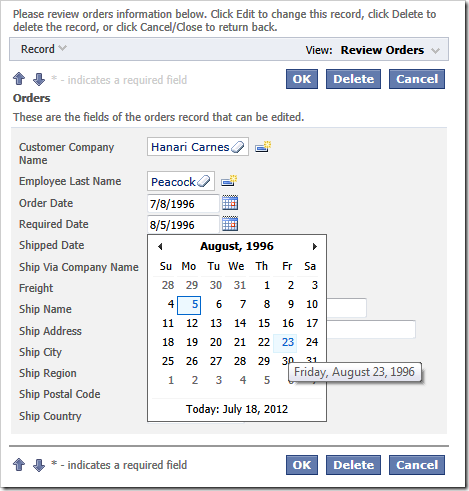
With a very little effort you can introduce an alternative calendar for all input elements rendered for the fields representing dates.
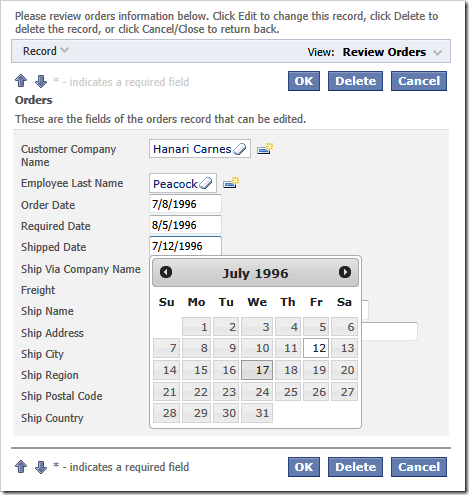
Start by enabling shared business rules in the application settings and re-generate the project.
Create the file [Documents]\Code OnTime\Client\Sample Editors\DatePicker.js and enter this script.
// The "factory" object for DateTime input elements
MyCompany$DatePicker = function () {
}
MyCompany$DatePicker.prototype = {
// This method is invoked for an input element of a data controller field
// associated with the editor. Return 'true' if no other editors are allowed.
attach: function (element, viewType) {
// hide the "calendar" button next to the input element
$(element).next().hide();
// attach a jQuery datepicker to the input element
$(element).datepicker();
return true;
},
// This method is invoked by the client library before the input element
// of the field is destroyed. Return 'true' if no other editors are allowed.
detach: function (element, viewType) {
// detach the datepicker from the input element
$(element).datepicker('destroy');
return true;
}
}
Start the code generator and the script will be integrated in the client library.
Activate Project Designer and click Browse to cause the application to be generated. Hit the browser Refresh button to ensure that the latest version of the client library is downloaded.
Switch to Project Designer and click Develop button on the toolbar. Visual Studio or Visual Web Developer will start. Open ~/App_Code/Rules/SharedBusinessRules.cs(vb) file and enter the following code.
C#:
using System;
using System.Data;
using System.Collections.Generic;
using System.Linq;
using MyCompany.Data;
namespace MyCompany.Rules
{
public partial class SharedBusinessRules : MyCompany.Data.BusinessRules
{
public override bool SupportsVirtualization(string controllerName)
{
return true;
}
protected override void VirtualizeController(string controllerName)
{
NodeSet().Select("field[@type='DateTime']").Attr("editor", "MyCompany$DatePicker");
}
}
}
Visual Basic:
Imports MyCompany.Data
Imports System
Imports System.Collections.Generic
Imports System.Data
Imports System.Linq
Namespace MyCompany.Rules
Partial Public Class SharedBusinessRules
Inherits MyCompany.Data.BusinessRules
Public Overrides Function SupportsVirtualization(controllerName As String) As Boolean
Return True
End Function
Protected Overrides Sub VirtualizeController(controllerName As String)
NodeSet().Select("field[@type='DateTime']").Attr("editor", "MyCompany$DatePicker")
End Sub
End Class
End Namespace
The methods of the shared business rules class will adjust the definition of data controllers with the help of virtualization node sets. The method VirtualizeController selects all date-time fields and assigns the custom editor at runtime.
Save the file and browse the application from the generator or directly in development environment. Date-time input elements in the entire app are now featuring a new calendar based on jQuery UI date picker.
Make sure to refresh the app when the first page is displayed in the web browser to ensure that the latest version of the custom scripts has been loaded.
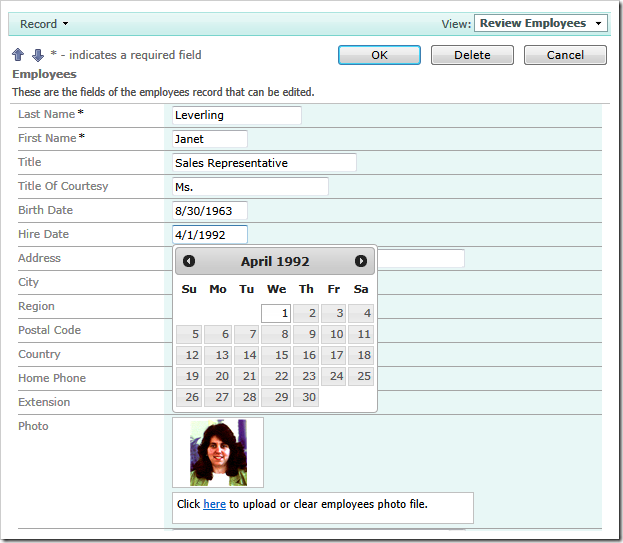