The core framework of your application always tries to create a class with the name YourProjectNamespace.Rules.SharedBusinessRules whenever it receives a request to process data on the server. The framework interacts with the class instance in order to determine if any custom business logic is implemented in the application. This happens when data is selected, updated, inserted, deleted, and when custom actions are requested.
The name YourProjectNamespace in the namespace entered in the project settings. The default namespace is MyCompany.
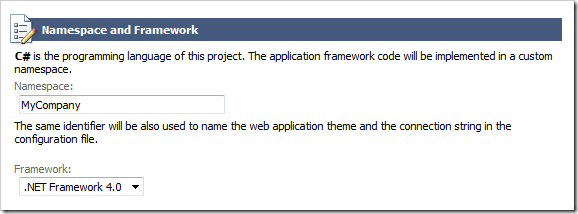
You can enable shared business rules if you select Settings of your project and choose the Business Logic Layer option. Toggle the check box in the Shared Business Rules section, click Finish button to save the changes.
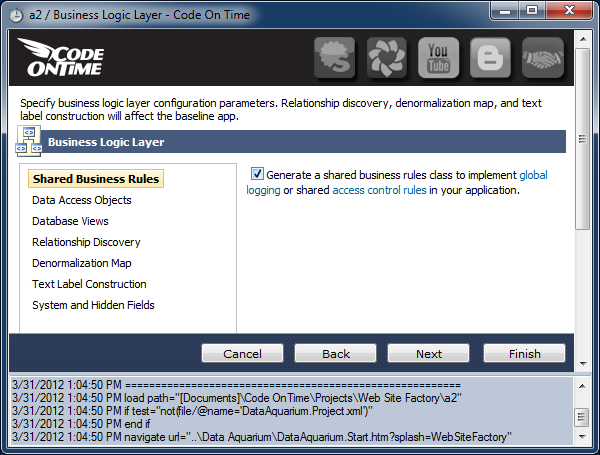
If you generate the app then the class file SharedBusinessRules.cs(vb) will be created either in ~/App_Code/Rules folder on in the ~/Rules folder of the application class library. This depends on the type of your projects.
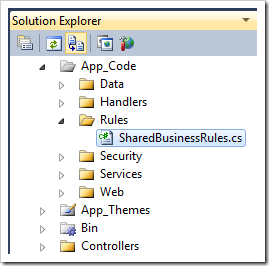
Class SharedBusinessRules provides a convenient placeholder for the business logic that may apply to all data controllers of your web application.
Enter the following code in the code file of shared business rules.
C#:
using System;
using System.Data;
using System.Collections.Generic;
using System.Linq;
using MyCompany.Data;
namespace MyCompany.Rules
{
public partial class SharedBusinessRules : MyCompany.Data.BusinessRules
{
[ControllerAction("", "grid1", "Select", ActionPhase.Before)]
protected void TellTheUserWhatJustHappened()
{
if (!IsTagged("UserIsInformed"))
{
Result.ShowMessage(
"You just have requested data from '{0}' controller",
ControllerName);
AddTag("UserIsInformed");
}
}
}
}
Visual Basic:
Imports MyCompany.Data
Imports System
Imports System.Collections.Generic
Imports System.Data
Imports System.Linq
Namespace MyCompany.Rules
Partial Public Class SharedBusinessRules
Inherits MyCompany.Data.BusinessRules
<ControllerAction("", "grid1", "Select", ActionPhase.Before)>
Protected Sub TellTheUserWhatJustHappened()
If (Not IsTagged("UserIsInformed")) Then
Result.ShowMessage(
"You just have requested data from '{0}' controller",
ControllerName)
AddTag("UserIsInformed")
End If
End Sub
End Class
End Namespace
Save the file and navigate to any page of your web application that contains data. Here is what you will see at the top of the page if you view the Suppliers in the Northwind sample.
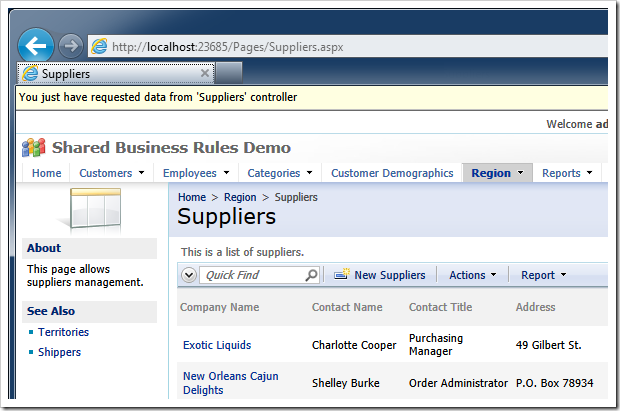
If you continue sorting, filter, and paging the suppliers then the message will continue to be displayed at the top. If you start editing or select a supplier then the message from the child view will popup.
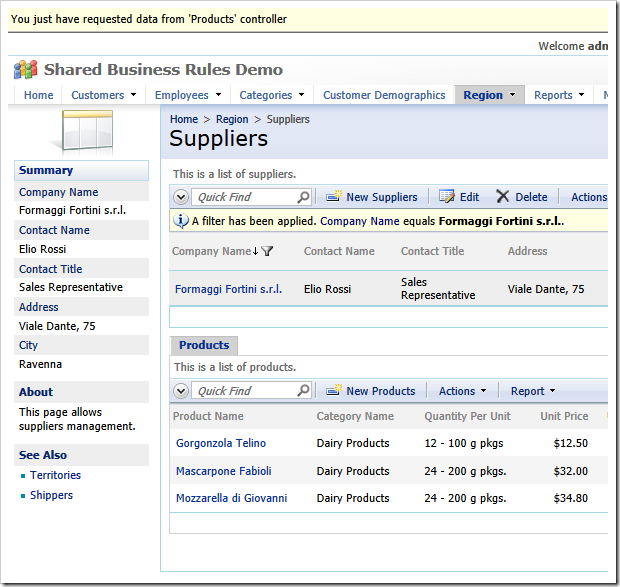
We are using IsTagged and AddTag methods to display the messages once in the lifecycle of the page instance in the browser window. If the data view on the page is not tagged then the message is displayed and the tag UserIsInformed is assigned to it.
The message will eventually disappear as soon as user invokes available data view actions.
Method TellTheUserWhatJustHappened is called every time the data is selected. This happens when the data is sorted, paged, changed, or if a filter is applied. Data view tagging ensures that the message will be sent to the client one time only.
If you navigate away from the page and come back using the Back button of your web browser then the tag is restored and no message will be displayed. If you click ahead and arrive to a non-historical version of the page then the data view tags will be blank and the message will show up once when the first attempt to select data is executed on the server.
The business logic implemented in this shared business rules example will be engaged for all data pages of your web application whenever the grid1 view of any controller requires data. This applies to an application with a single data page and will also be true in the application with three hundred data pages.
If you have existing controller-specific business rules, then make sure that their base class is changed from BusinessRules to SharedBusinessRules. If you create new controller-specific business rules, then the code generator will automatically specify the SharedBusinessRules class as their base.