Implementation of input element enhancements may be very simple or demand a relatively complex code. The client input elements are written in JavaScript and may require debugging.
The first instinct of any developer is to write some code, run the app, and try the code in action. Developing and debugging a custom input element with the entire web application project may become unproductive – you need to sign in, navigate to the correct page, select a view to force the custom input element to be activated. Each step takes valuable time.
We recommend creating a test HTML page and debugging the app without touching the actual application.
Let’s consider creating a test HTML page for the Reorder Level Slider input element shown in this screen shot of a live application.
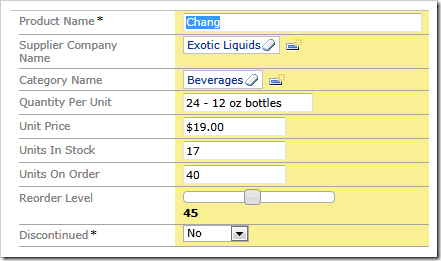
If you have followed the instruction from the tutorial then you have the following folder structure under [Documents]\Code On Time\Client folder.
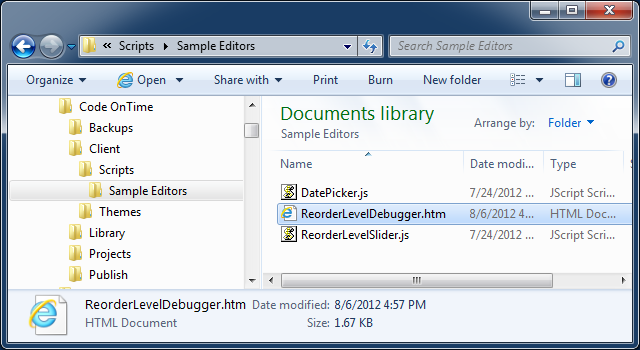
Create the HTML file ReorderLevelDebugger.htm with the following content in Visual Studio or your favorite text editor.
<!DOCTYPE html PUBLIC "-//W3C//DTD XHTML 1.0 Transitional//EN"
"http://www.w3.org/TR/xhtml1/DTD/xhtml1-transitional.dtd">
<html xmlns="http://www.w3.org/1999/xhtml">
<head>
<title>Edtior Debugger</title>
<link type="text/css" rel="Stylesheet" href="../../../Library/_Client/Stylesheets/_System/_System.css" />
<script type="text/javascript" src="../../../Library/Data Aquarium/Scripts/_System.js"></script>
<!--
Important:
link the file(s) that you are debugging after the reference to _System.js
-->
<script type="text/javascript" src="ReorderLevelSlider.js"></script>
<script type="text/javascript">
// this code is the emulation of steps executed by the client library
var reorderLevelEditorFactory = null;
$(function () {
// create a slider factory object
reorderLevelEditorFactory = new MyCompany$ReorderLevelSlider()
// initialize the debugging buttons
$('#Attach').click(function () {
reorderLevelEditorFactory.attach($('#Input1').get(), 'Form');
});
$('#Detach').click(function () {
reorderLevelEditorFactory.detach($('#Input1').get(), 'Form');
});
});
</script>
</head>
<body>
<div>
Testing the "Reorder Level" slider:
</div>
<div>
<button id="Attach">
Attach</button>
<button id="Detach">
Detach</button></div>
<div style="border: solid 1px silver; padding: 8px; margin-top: 8px;">
<input type="text" id="Input1" value="35" />
</div>
</body>
</html>
The file declares a script block with the global instance of MyCompany$ReorderLevelSlider object. The startup script also assigns click handlers for Attach and Detach buttons declared in the page body. The script header links the system script and stylesheet included by the code generator in every application.
Save the file and open it in the web browser. This is how the page is rendered in Internet Explorer 9 if you click on the file name in Windows Explorer.
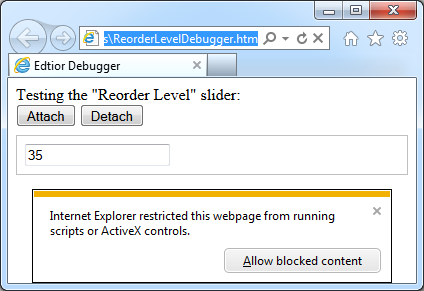
You will need to allow execution of JavaScript in the local webpage. This will not be necessary if you preview the page by selecting View in Browser (Ctrl+Shift+W) in the Visual Studio development environment.
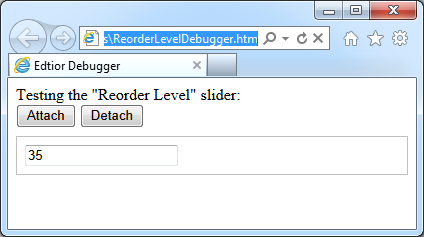
Click Attach button to see the slider attached to the input field.
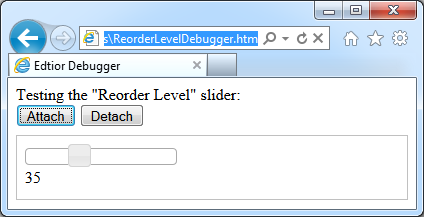
Move the slider to try it out.
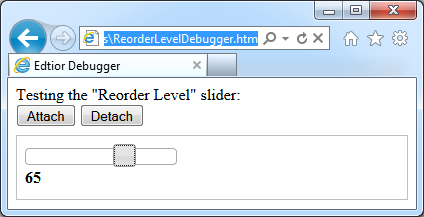
Click Detach button to see the slider removed.
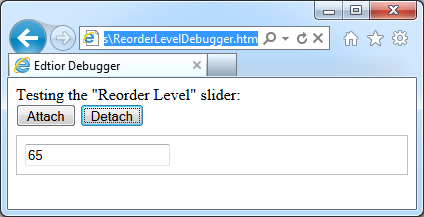
Buttons Attach and Detach invoke the corresponding methods of the “factory” object to associate custom input enhancement with the input field and to have them removed. This is exactly the same pattern of execution by the client library in the live web application.