The client library of a generated web application offers a tightly integrated suite of presentation views and input controls with automatic data binding. The library is not designed to replace the popular presentation frameworks such as jQuery or Ajax Control Toolkit. The purpose of the client library is to provide a standard extensible presentation layer of a multi-tier web application.
Consider the following screen of the Northwind sample. The standard input elements lookup, dropdown, and text are rendered in the Products form view. It is possible to replace the standard text input element of the Reorder Level field with a modern input control.
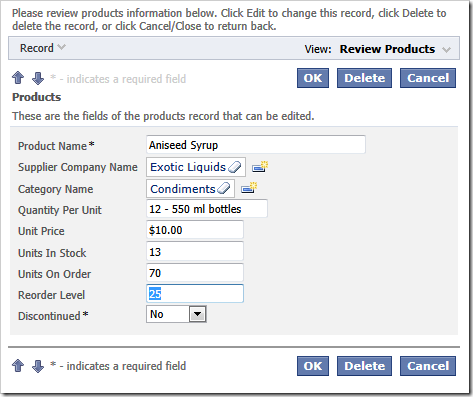
The application client library incorporates the entire jQuery and jQuery UI. This allows using a slider plugin for presentation purposes.
The screenshot shows the slider input control in action.
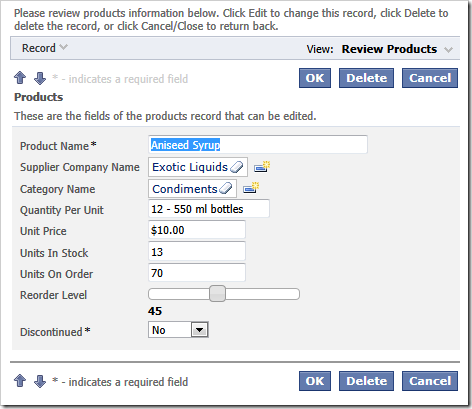
Input controls are implemented in JavaScript language.
Custom scripts are combined in a single file _System.js, which is included in each generated application. The code generator will automatically build this file from the scripts located under [Documents]\Code OnTime\Client\Scripts folder. The generator scans the folder every time it starts and tries to detect any changes to scripts located there. If the changes are detected, then the entire set of scripts is combined and stored in the single [Documents]\Code OnTime\Library\Data Aquarium\Scripts\_System.js file. The combined script is copied to the appropriate output folder of the project when the app is generated.
Here is how the scripts may be organized on the hard drive of your computer.
![Sample contents of '[Documents]\Code OnTime\Client' folder. Sample contents of '[Documents]\Code OnTime\Client' folder.](/blog/2012/07/enhancing-applications-with-custom/image06.png)
Close the code generator and create the file [Documents]\Code OnTime\Client\Scripts\Sample Editors\ReorderLevelSlider.js using Visual Studio or your favorite text editor. Enter the following content and save the file.
// The "factory" object for Reorder Level input field
MyCompany$ReorderLevelSlider = function () { }
MyCompany$ReorderLevelSlider.prototype = {
// This method is invoked for an input element of a data controller field
// associated with the editor. Return 'true' if no other editors are allowed.
attach: function (element, viewType) {
if (viewType != 'Form')
return false;
// figure the initial Reorder Level
var reorderLevel = $(element).val();
reorderLevel = reorderLevel == '' ? 0 : parseInt(reorderLevel);
// mark the element and its parent with CSS classes and hide the element
$(element)
.hide().addClass('reorder-level-elem')
.parent().addClass('reorder-level-parent');
// create a 'div' element with a slider
var slider = $('<div>').insertAfter(element)
.width(150)
.css({ marginTop: '4px', marginBottom: '4px' })
.slider({
value: reorderLevel,
min: 0,
max: reorderLevel < 100 ? 100 : reorderLevel,
step: 5,
slide: function (event, ui) {
// find the parent element of the ReorderLevel input
var parent = $(ui.handle).parents('.reorder-level-parent');
// change the value of the ReorderLevel input
$('.reorder-level-elem', parent).val(ui.value);
// update the label with the value of the slider
$('.reorder-level-label', parent).text(ui.value)
.css('font-weight', 'bold');
}
});
// create a 'span' to be used as a slider label
$('<div>').insertAfter(slider)
.text($(element).val())
.addClass('reorder-level-label')
.css('marginBottom', '4px');
return true;
},
// This method is invoked by the client library before the input element
// of the field is destroyed. Return 'true' if no other editors are allowed.
detach: function (element, viewType) {
if (viewType != 'Form')
return false;
// detach the slider from the input element
$(element).siblings('div:ui-slider').slider('destroy'); // mandatory
$(element).siblings('span,div').remove(); // optional cleanup
$(element).show(); // optional call
return true;
}
}
The script defines a “factory” object MyCompany$ReorderLevelSlider that will be invoked by the client library to allow attaching and detaching custom input enhancements at runtime for the designated data controller fields. Methods attach and detach must return true if no other enhancements are allowed to be associated with the input field. The client library will create a single instance of a “factory” object and use is it as needed when the presentation interface of a web app is changed in response to user actions. If input elements are rendered then the factory object will be asked to attach to the inputs associated with it. If the input elements are not needed anymore then the client library will ask the “factory” object to remove any enhancements it may have created previously.
Start the web application generator. The new script will become a part of the _System.js file. It can now be used in any project that you are working on.
Activate Project Designer and select Controllers / Products / Fields / ReorderLevel field node on the Controllers tab of Project Explorer.
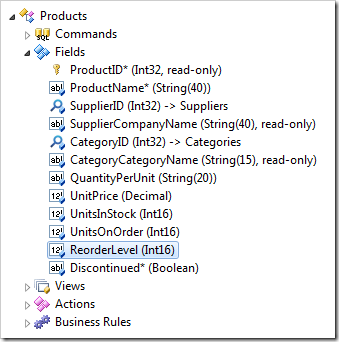
Change the field as follows.
Property |
New Value |
Editor |
MyCompany$ReorderLevelSlider |
Save the changes, click Browse button on the designer toolbar.
Make sure to refresh the application as soon as any of its pages in displayed in the browser to ensure that the most recent version of the _System.js file is downloaded.
Navigate to the Products page, select a product and start editing in a form view. The new input control will be displayed.