A dedicated “Status” field in a database table is a simple and easy to implement method of separating “draft” and “committed” data. For example, orders stored in a database will be explicitly marked with a status of “Committed” if no changes are allowed to them. Orders still being composed will be marked with a status of “Draft”. The application must ignore draft data and have it visible only on the order entry page.
Adding the “Status” Column
Start SQL Server Management Studio. In the Object Explorer, right-click on Databases / Northwind and press New Query.
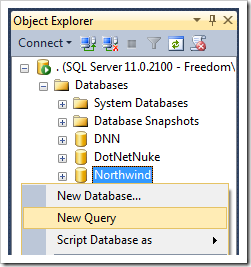
Paste in the following script to add the table column and mark all existing orders as “Committed”.
alter table Orders
add Status nvarchar(50) default 'Draft'
go
update Orders
set Status = 'Committed'
go
On the toolbar, press Execute to run the query.
Controlling Display of Draft Orders
Start the web application generator. Select the project name and click Settings. Press Business Logic Layer and enable shared business rules. Click Finish and regenerate the project.
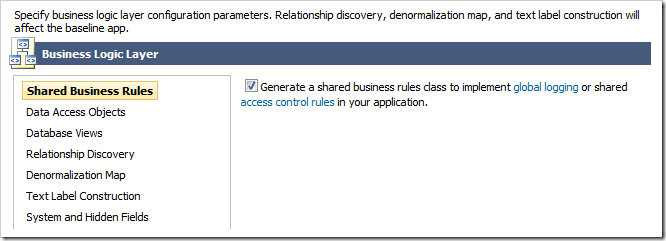
Start the Project Designer. In the Project Explorer, switch to the Controllers tab. Right-click on Orders controller node, and press Edit Handler in Visual Studio.
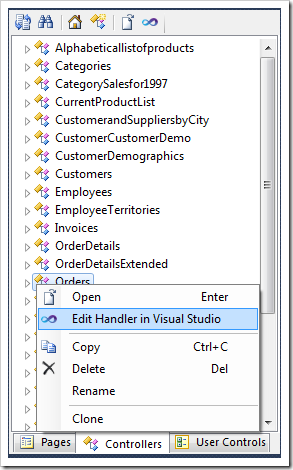
The shared business rule file will open in Visual Studio. Replace the file with the following code:
C#:
using System;
using System.Data;
using System.Collections.Generic;
using System.Linq;
using MyCompany.Data;
namespace MyCompany.Rules
{
public partial class SharedBusinessRules : MyCompany.Data.BusinessRules
{
public SharedBusinessRules()
{
}
protected override void EnumerateDynamicAccessControlRules(string controllerName)
{
if (Context.Request.UrlReferrer != null)
{
if (Context.Request.UrlReferrer.ToString().ToLower().Contains("orderform.aspx"))
RegisterAccessControlRule("OrderID",
"select OrderID from Orders where Status = 'Draft'",
AccessPermission.Allow);
else
RegisterAccessControlRule("OrderID",
"select OrderID from Orders where Status = 'Committed'",
AccessPermission.Allow);
}
}
}
}
Visual Basic:
Imports MyCompany.Data
Imports System
Imports System.Collections.Generic
Imports System.Data
Imports System.Linq
Namespace MyCompany.Rules
Partial Public Class SharedBusinessRules
Inherits MyCompany.Data.BusinessRules
Public Sub New()
MyBase.New()
End Sub
Protected Overrides Sub EnumerateDynamicAccessControlRules(controllerName As String)
If Context.Request.UrlReferrer <> Nothing Then
If Context.Request.UrlReferrer.ToString().ToLower().Contains("orderform.aspx") Then
RegisterAccessControlRule("OrderID",
"select OrderID from Orders where Status = 'Draft'",
AccessPermission.Allow)
Else
RegisterAccessControlRule("OrderID",
"select OrderID from Orders where Status = 'Committed'",
AccessPermission.Allow)
End If
End If
End Sub
End Class
End Namespace
The implementation will conditionally register a dynamic access control rule that will apply to a view of any data controller with an OrderID data field. If the user is interacting with an application page ~/Pages/OrderForm.aspx, then only data that matches orders with a status of “Draft” is allowed. Otherwise, only data that matches orders with a status of “Committed” is included in the returned data set.
Adding “Submit Order” Action
In the Project Explorer, right-click on Orders / Actions / ag2 (Form) node, and press New Action.
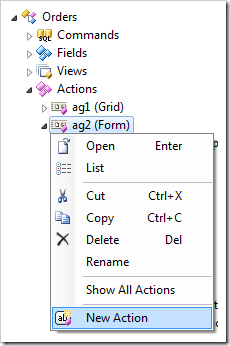
Assign the following values:
Property |
Value |
Command Name |
Custom |
Command Argument |
SubmitOrder |
Header Text |
Submit Order |
Press OK to save the action. Drop Orders / Actions / ag2 (Form) / a101 – Custom, SubmitOrder | Submit Order node on the left side of a100 – Report | Order Report to place it first.
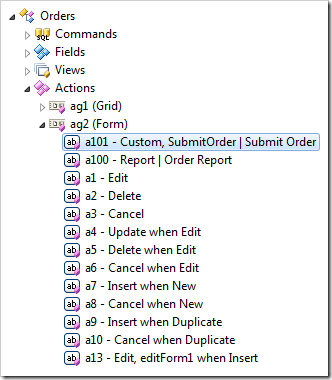
Adding Business Rule
Right-click on Orders / Business Rules node, and press New Business Rule.
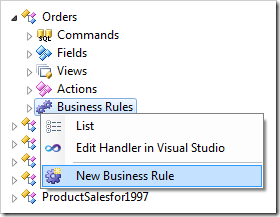
Assign these values:
Property |
Value |
Type |
SQL |
Command Name |
Custom |
Command Argument |
SubmitOrder |
Phase |
Execute |
Script |
update Orders
set Status = 'Committed'
where OrderID = @OrderID
set @Result_NavigateUrl = 'OrderForm.aspx'
|
The business rule will set Status column of the selected order to “Committed” and refresh the page loaded in the web browser. Press OK to save.
Testing the Results
On the toolbar, press Browse. Navigate to the Order Form page. Create a new order and return to the grid. Note that only a single draft order is listed.
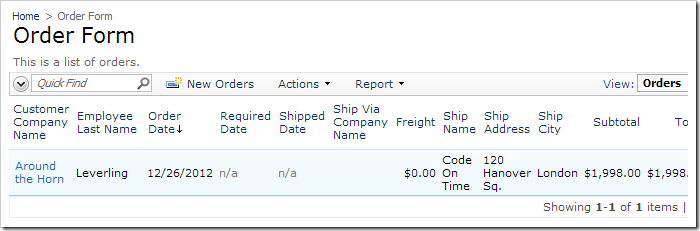
Navigate to the Orders page. Note that the all of the orders are displayed except for the draft order.
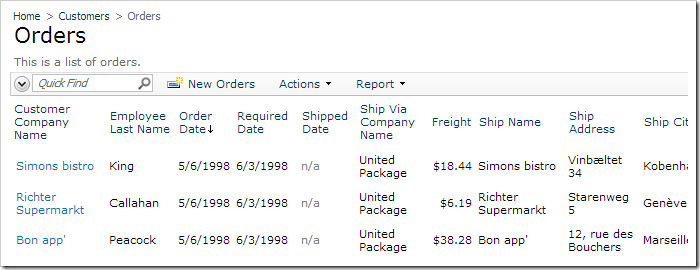
The draft order will also not be visible in any data controllers based on database views if they relate to orders. For example, page Reports | Order Subtotals will not display the new order. The dynamic access control rule explained above filters the order out.
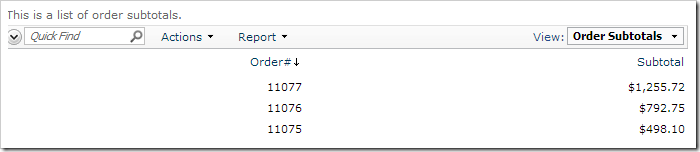
Go back to the Order Form page, and select the draft order. Click on the “Submit Order” button.
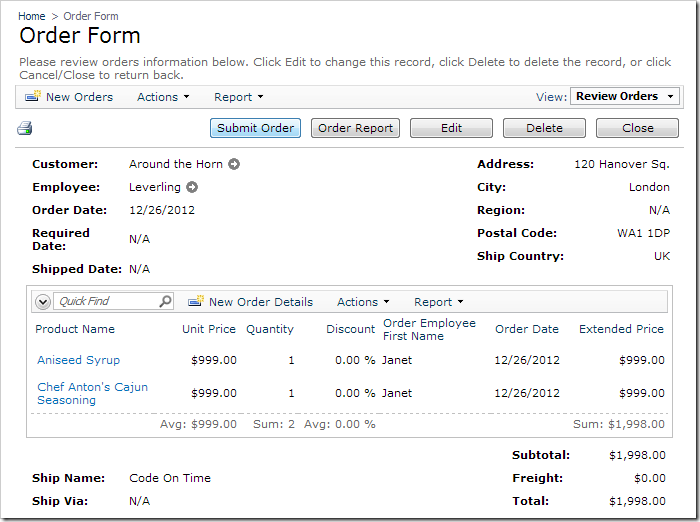
The browser will refresh the page and display an empty list of orders.
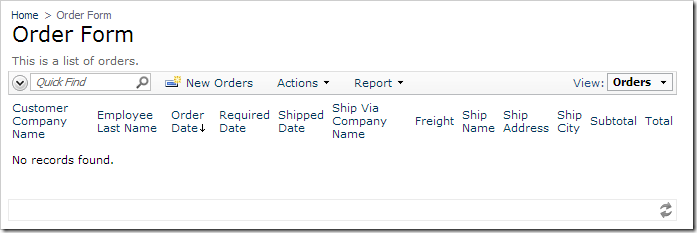
The order will now be displayed on Orders page.
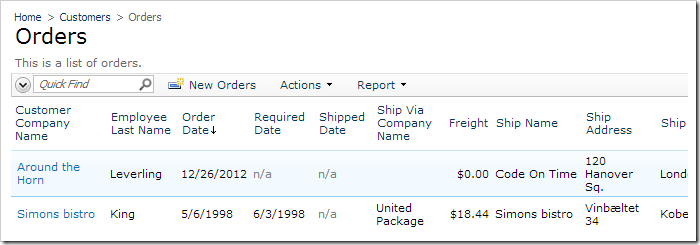
It will also be displayed on Order Subtotals page that can be found under the Reports option in the application navigation menu.
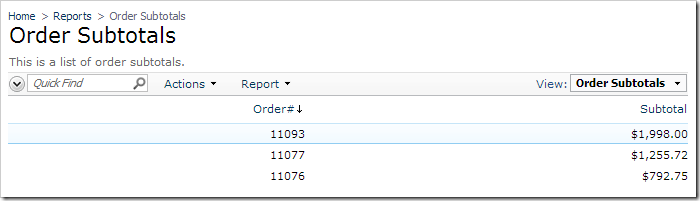