Business requirements can change hourly in the world of broadband connections and instant messages . Your application will have to be changed no matter how good you are at putting together a detailed requirements specification for the project. Some obscure security rules will be missed, new clients will require special handling – you know the story.
It is virtually impossible to create an application that will adapt to changing security and data segregation requirements unless you are building your web applications with Code On Time.
The unique architecture of generated web applications allows dynamic engagement of access control rules without changing a single line in the source code of your project.
Consider the following screen shot of the Northwind sample with a seemingly random list of ten customer accounts.
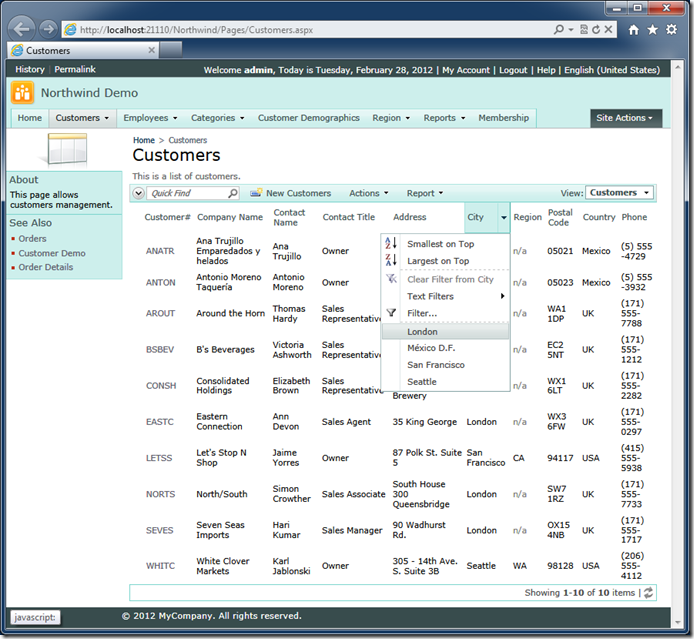
The actual web application source code defines a business rules class with an override of EnumerateDynamicAccessControlRules method. This method registers a few access control rules whenever the customer accounts are retrieved from the application database.
Here is the code.
C#:
using System;
using System.Data;
using System.Collections.Generic;
using System.Linq;
using MyCompany.Data;
namespace MyCompany.Rules
{
public partial class CustomersBusinessRules : MyCompany.Data.BusinessRules
{
protected override void EnumerateDynamicAccessControlRules(string controllerName)
{
RegisterAccessControlRule(
"CustomerID", AccessPermission.Allow, "ANTON", "ANATR");
RegisterAccessControlRule(
"CustomerID",
"[Country]=@Country and [ContactTitle] = @ContactTitle",
AccessPermission.Allow,
new SqlParam("@Country", "USA"),
new SqlParam("@ContactTitle", "Owner"));
RegisterAccessControlRule(
"CustomerID",
"select CustomerID from Customers " +
"where Country=@Country2 and City=@City",
AccessPermission.Allow,
new SqlParam("@Country2", "UK"),
new SqlParam("@City", "London"));
}
}
}
Visual Basic:
Imports MyCompany.Data
Imports System
Imports System.Collections.Generic
Imports System.Data
Imports System.Linq
Imports System.Xml
Imports System.Xml.XPath
Namespace MyCompany.Rules
Partial Public Class CustomersBusinessRules
Inherits MyCompany.Data.BusinessRules
Protected Overrides Sub EnumerateDynamicAccessControlRules(controllerName As String)
RegisterAccessControlRule(
"CustomerID", AccessPermission.Allow, "ANTON", "ANATR")
RegisterAccessControlRule(
"CustomerID",
"[Country]=@Country and [ContactTitle] = @ContactTitle",
AccessPermission.Allow,
New SqlParam("@Country", "USA"),
New SqlParam("@ContactTitle", "Owner"))
RegisterAccessControlRule(
"CustomerID",
"select CustomerID from Customers " +
"where Country=@Country2 and City=@City",
AccessPermission.Allow,
New SqlParam("@Country2", "UK"),
New SqlParam("@City", "London"))
End Sub
End Class
End Namespace
Let’s review the effect of each individual rule on our data set.
The first call of RegisterAccessControlRule method limits a list of customers to those with the specific customer IDs of ANTON and ANATR.
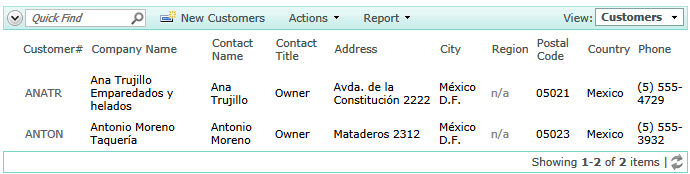
The second call limits a list of customers to those from the USA and having the title of Owner.
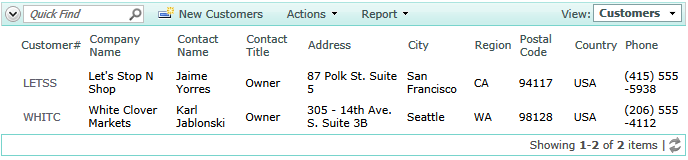
The third call limits a list of customers to those from the United Kingdom and located in the city of London.
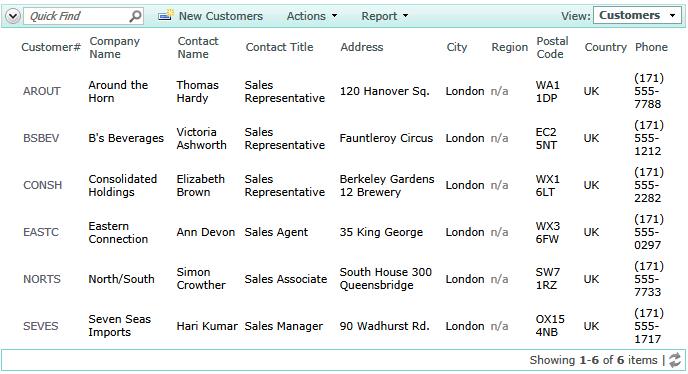
The three calls are showcasing various methods of creating a dynamic restriction.
The final result is a cumulative set of 10 records since the rules do not contradict each other.
The access control rules used in the example are registered unconditionally and hard-coded in the application business logic.
What if you take this rules and store them in the database? The rules can be loaded on-demand based on the user identity and injected in the application without changing anything at all. Better yet, you can enable shared business rules in your project and have only one implementation of EnumerateDynamicAccessControlRules method.
We leave the actual implementation up to you.
If a custom implementation of dynamic access control rules is too much for you or is simply not required then continue using the static access control rules.