Integration with other service providers is a key to success of many SaaS business applications. The mail order company Northwind Traders has decided to expose their product catalog to third parties to increase sales. A back office web app has been created with Code On Time and configured to expose the product catalog.
An integration partner can put together a simple HTML page to display a list of products from http://demo.codeontime.com/northwind with a very few lines of code.
<!DOCTYPE html>
<html xmlns="http://www.w3.org/1999/xhtml">
<head>
<title>jQuery Cross-Domain Client</title>
<script src="http://ajax.googleapis.com/ajax/libs/jquery/1.8.1/jquery.min.js"></script>
<script src="http://demo.codeontime.com/northwind/appservices/Products?_instance=ProductCatalog">
</script>
<script type="text/javascript">
$(document).ready(function () {
$.each(MyCompany.ProductCatalog.Products, function (index, product) {
$('<option>')
.text(
product.ProductName + ' / ' +
product.CategoryCategoryName + ' / ' +
product.SupplierCompanyName + ' / ' +
product.UnitPrice)
.attr('value', product.ProductID)
.appendTo($('#ProductList'));
});
});
</script>
</head>
<body>
<select id="ProductList" size="20">
</select>
</body>
</html>
The first script tag links jQuery library to the page straight from the Google’s CDN.
The second script references http://demo.codeontime.com/northwind/appservices/Products. This URL points to the demo web app. The highlighted component /appservices/Products references the REST URI of the Products data controller. The _instance parameter instructs the application to construct a JavaScript property with the name of ProductCatalog. The property will be assigned to MyCompany global variable that matches the namespace of the application.
The script executes as soon as the page has been loaded in the browser and iterates through MyCompany.ProductCatalog.Products array to populate the select element ProductList defined in the page body.
This is how the page will look if you have it opened in your default web browser.
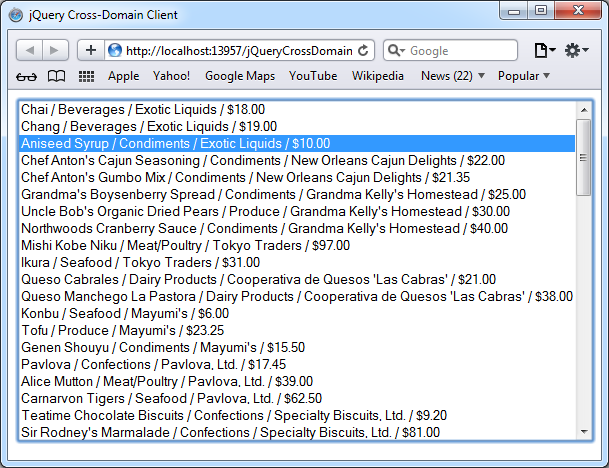
If you open the page straight from the hard drive in Internet Explorer, then you many need to allow running scripts for the list of products to get displayed.
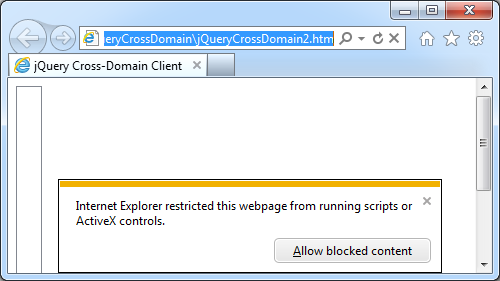
The product catalog is dynamically constructed by the web app every time it is requested.
This is how the array of products look in debug mode if global variable MyCompany is inspected in Visual Studio.
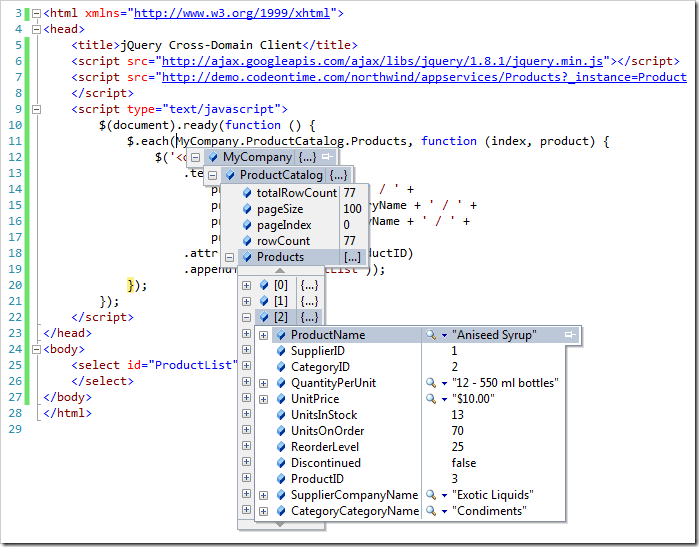
If more than one resource pointing to the demo app controller is linked to the page then data will be available as properties of MyCompany global variable.
The Product Catalog data is downloaded by a web browser from the demo web app regardless of the domain of the HTML page or web platform that has served the page. This opens tremendous opportunities of integrating data in the third-party solutions.