It may be necessary to copy similar values from a lookup to a record.
For simple copy operations, the Copy property can be used. For more complex operations, a business rule can be implemented. Let’s implement a C# / Visual Basic business rule to copy shipping information from a customer to an order in a sample Northwind web application.
Start the Project Designer. In the Project Explorer, switch to the Controllers tab and double-click on Orders / Fields / ShipName node.
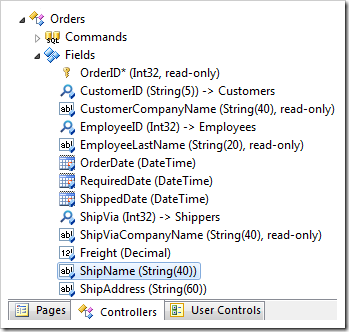
Change the following propeties.
Property | Value |
The value of the field is calculated by a business rule expression | true |
Context Fields | CustomerID |
Press OK to save the field.
Right-click on Orders / Business Rules node and press New Business Rule.
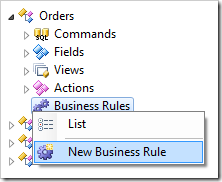
Give this rule the following properties.
Property | Value |
Type | C# / Visual Basic |
Command Name | New|Calculate |
Phase | Execute |
Press OK to save the business rule. On the toolbar, press Browse to generate the web application and business rule file.
When complete, right-click on Orders / Business Rules / New|Calculate node, and press Edit Rule in Visual Studio.
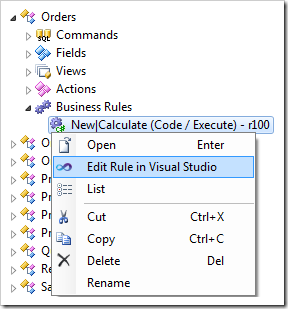
The business rule handler will open in Visual Studio. Replace the default implementation with the following:
C#:
using System;
using MyCompany.Data;
namespace MyCompany.Rules
{
public partial class OrdersBusinessRules : MyCompany.Data.BusinessRules
{
/// <summary>
/// This method will execute in any view for an action
/// with a command name that matches "New|Calculate".
/// </summary>
[Rule("r100")]
public void r100Implementation(
int? orderID,
string customerID,
string customerCompanyName,
int? employeeID,
string employeeLastName,
DateTime? orderDate,
DateTime? requiredDate,
DateTime? shippedDate,
int? shipVia,
string shipViaCompanyName,
decimal? freight,
string shipName,
string shipAddress,
string shipCity,
string shipRegion,
string shipPostalCode,
string shipCountry)
{
// This is the placeholder for method implementation.
if (!String.IsNullOrEmpty(customerID))
using (SqlText findCustomer = new SqlText(
"select " +
" ContactName, " +
" Address, " +
" City, " +
" Region, " +
" PostalCode, " +
" Country " +
"from Customers " +
"where " +
" CustomerID = @CustomerID "))
{
findCustomer.AddParameter("@CustomerID", customerID);
if (findCustomer.Read())
{
UpdateFieldValue("ShipName", findCustomer["ContactName"]);
UpdateFieldValue("ShipAddress", findCustomer["Address"]);
UpdateFieldValue("ShipCity", findCustomer["City"]);
UpdateFieldValue("ShipRegion", findCustomer["Region"]);
UpdateFieldValue("ShipPostalCode", findCustomer["PostalCode"]);
UpdateFieldValue("ShipCountry", findCustomer["Country"]);
}
}
}
}
}
Visual Basic:
Imports MyCompany.Data
Imports System
Namespace MyCompany.Rules
Partial Public Class OrdersBusinessRules
Inherits MyCompany.Data.BusinessRules
''' <summary>
''' This method will execute in any view for an action
''' with a command name that matches "New|Calculate".
''' </summary>
<Rule("r100")> _
Public Sub r100Implementation( _
ByVal orderID As Nullable(Of Integer), _
ByVal customerID As String, _
ByVal customerCompanyName As String, _
ByVal employeeID As Nullable(Of Integer), _
ByVal employeeLastName As String, _
ByVal orderDate As Nullable(Of DateTime), _
ByVal requiredDate As Nullable(Of DateTime), _
ByVal shippedDate As Nullable(Of DateTime), _
ByVal shipVia As Nullable(Of Integer), _
ByVal shipViaCompanyName As String, _
ByVal freight As Nullable(Of Decimal), _
ByVal shipName As String, _
ByVal shipAddress As String, _
ByVal shipCity As String, _
ByVal shipRegion As String, _
ByVal shipPostalCode As String, _
ByVal shipCountry As String)
'This is the placeholder for method implementation.
If Not String.IsNullOrEmpty(customerID) Then
Using findCustomer As SqlText = New SqlText(
"select " +
" ContactName, " +
" Address, " +
" City, " +
" Region, " +
" PostalCode, " +
" Country " +
"from Customers " +
"where " +
" CustomerID = @CustomerID ")
findCustomer.AddParameter("@CustomerID", customerID)
If (findCustomer.Read()) Then
UpdateFieldValue("ShipName", findCustomer("ContactName"))
UpdateFieldValue("ShipAddress", findCustomer("Address"))
UpdateFieldValue("ShipCity", findCustomer("City"))
UpdateFieldValue("ShipRegion", findCustomer("Region"))
UpdateFieldValue("ShipPostalCode", findCustomer("PostalCode"))
UpdateFieldValue("ShipCountry", findCustomer("Country"))
End If
End Using
End If
End Sub
End Class
End Namespace
Save the file. Switch back to the Project Designer, and press Browse on the toolbar.
Navigate to Customers page. Select a customer, and a list of orders will appear underneath. On the action bar, press New Orders. The shipping information will be populated using the customer information.
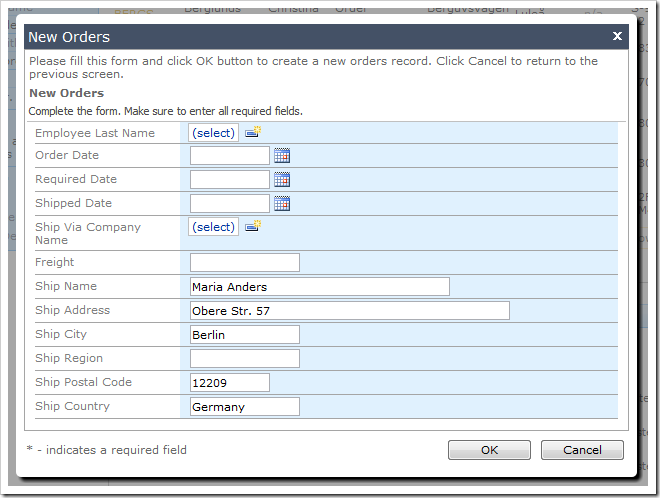
Navigate to the Orders page. On the action bar, press New Orders. The shipping fields will be empty.
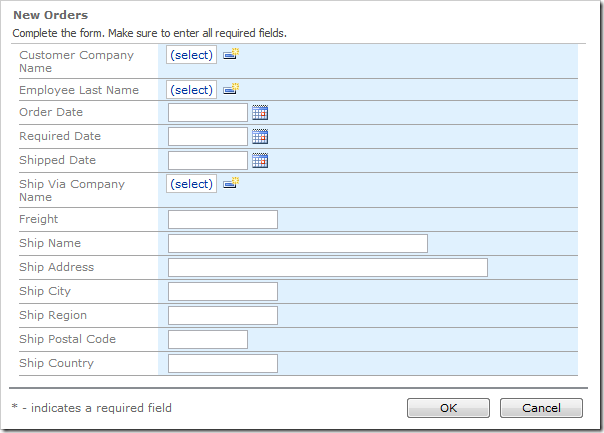
Select a customer using the Customer Company Name lookup. The shipping information will be populated.
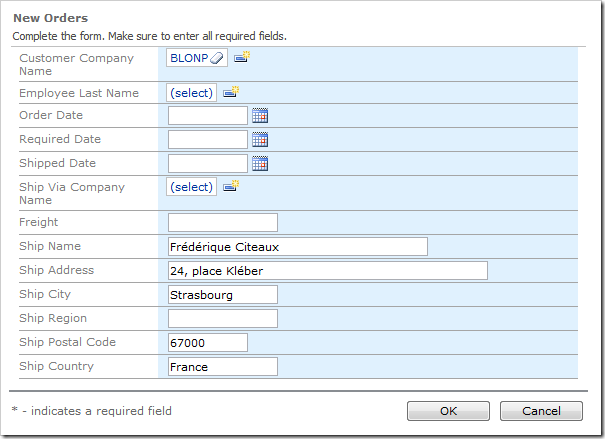