Suppose that you want to change a field that renders as a textbox into a static drop down list of items, such as the Reorder Level field in the Products controller of the Northwind sample. By default, users can enter any numeric value in the field.
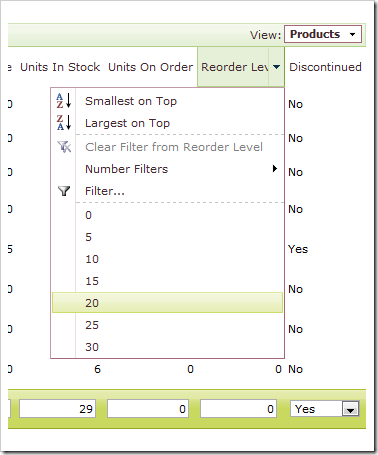
Let’s change this by offering a drop down list of predefined items, and have the item values displayed as words.
You can do this at design time, as explained in Creating Static Lookup Items. However, there may be other situations when you don’t want to change the physical properties of the application. For example, the static items maybe displayed only to users in specific roles. For this purpose, you can use virtualization node sets.
First, you will need to enable shared business rules.
Next, switch to the web application generator, select the project name, and press Develop. This will open the project in Visual Studio.
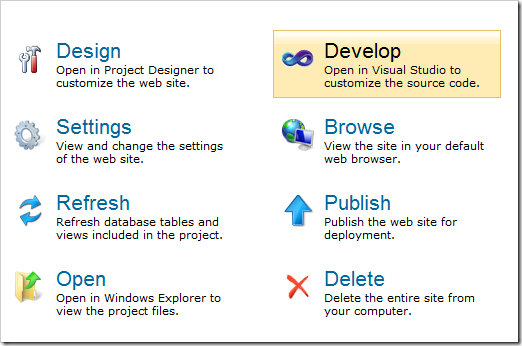
In the Solution Explorer, navigate to ~/App_Code/Rules/SharedBusinessRules.cs(vb). The code will need to enable virtualization if the controller name is “Products”. The virtualized Products controller will have a modified ReorderLevel field. The Items Style will be changed to “Drop Down List”, and it will have the following static items:
Value | Text |
0 | Zero |
5 | Five |
10 | Ten |
15 | Fifteen |
20 | Twenty |
25 | Twenty-five |
30 | Thirty |
Place the code into your SharedBusinessRules.cs(vb) file:
C#:
using System;
using System.Data;
using System.Collections.Generic;
using System.Linq;
using MyCompany.Data;
namespace MyCompany.Rules
{
public partial class SharedBusinessRules : MyCompany.Data.BusinessRules
{
public override bool SupportsVirtualization(string controllerName)
{
if (controllerName == "Products")
return true;
return false;
}
protected override void VirtualizeController(string controllerName)
{
if (controllerName == "Products")
{
NodeSet().SelectField("ReorderLevel")
.SetItemsStyle("DropDownList")
.CreateItem(0, "Zero")
.CreateItem(5, "Five")
.CreateItem(10, "Ten")
.CreateItem(15, "Fifteen")
.CreateItem(20, "Twenty")
.CreateItem(25, "Twenty-five")
.CreateItem(30, "Thirty");
}
}
}
}
Visual Basic:
Imports MyCompany.Data
Imports System
Imports System.Collections.Generic
Imports System.Data
Imports System.Linq
Namespace MyCompany.Rules
Partial Public Class SharedBusinessRules
Inherits MyCompany.Data.BusinessRules
Public Overrides Function SupportsVirtualization(controllerName As String) As Boolean
If (controllerName = "Products") Then
Return True
Else
Return False
End If
End Function
Protected Overrides Sub VirtualizeController(controllerName As String)
If (controllerName = "Products") Then
NodeSet().SelectField("ReorderLevel") _
.SetItemsStyle("DropDownList") _
.CreateItem(0, "Zero") _
.CreateItem(5, "Five") _
.CreateItem(10, "Ten") _
.CreateItem(15, "Fifteen") _
.CreateItem(20, "Twenty") _
.CreateItem(25, "Twenty-five") _
.CreateItem(30, "Thirty")
End If
End Sub
End Class
End Namespace
Save this file and run the web application. You will see that the Products controller now displays Reorder Level filter options as words, and the field is rendered as a drop down list.
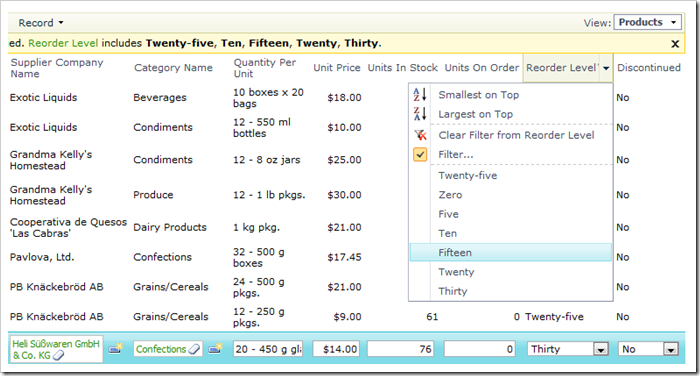