The workflow dashboard tutorial explains data controller virtualization.
Implementation of method VirtualizeController creates virtualization node sets to alter the data controller XML definition.
For example, the following snippet sorts the grid view grid1 in descending order of “Required Date” and rearranges its data fields by making “ShippedDate”, “EmployeeID”, and “ShipCity” appear first. The code snippet is written in Visual Basic.
If (IsTagged("OrdersShippedLate")) Then
' sort and rearrange 'grid1' by placing ShippedDate, EmployeeID, and ShipCity first
NodeSet("view[@id='grid1']") _
.Attr("sortExpression", "RequiredDate desc") _
.Select("dataField") _
.Arrange("@fieldName", "ShippedDate", "EmployeeID", "ShipCity")
End If
This is the data view without the virtualization node set:
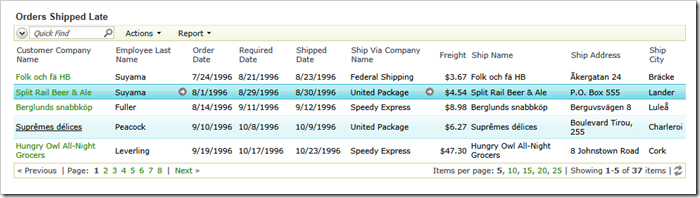
This is the result of the virtualization node set executed at runtime:
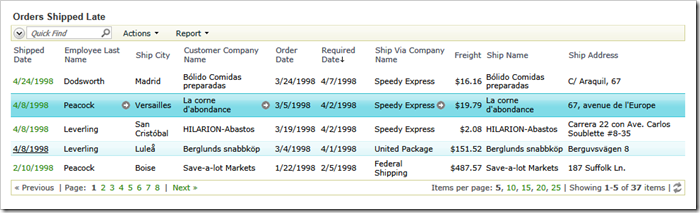
Virtualization node sets alter the in-memory copy of the data controller XML definition. The in-memory copy is discarded as soon as the request from the data view has been processed.
This allows treating the entire application as a large toolkit full of building blocks. Each block may allow a maximum set of capabilities.
Developer uses virtualization node sets when implementing virtualization rules to change the building blocks. The data controller elements can be removed or changed in an arbitrary fashion. For example, the virtualization node set can be executed if a certain use case of the data controller is detected. The user identity may also trigger the virtualization node set execution.
Developers can also elect to add new capabilities to the data controller that were not defined at design time.
The example above uses the method BusinessRules.NodeSet() to create the virtualization node set. Chained calls of Attr(), Select(), and Arrange() methods are altering the data controller XML definition. Each method works on the collection of XML nodes produced by the previous call in the chain.
The basic virtualization node set methods allow selecting, deleting, arranging, and changing attributes and elements of the XML document. Selector expressions written in XPath are used as arguments of the basic methods.
The collection of named plugins is also available. The specially named methods of the virtualization node sets make the node sets much easier to understand. They do not require knowledge or understanding of XPath. Instead the methods are named after the familiar logical elements that developers are modifying in the Project Designer.
The following implementations of method VirtualizeController are written with the use of “plugin” methods instead of the basic ones. Basic methods are shown in the workflow dashboard tutorial. Each virtualization node set starts with the call to method NodeSet() without parameters and continues with calls of “named plugin” methods altering specific areas of the data controller.
C#:
protected override void VirtualizeController(string controllerName)
{
if (controllerName == "Orders" && IsTagged("OrdersWaitingToShip", "OrdersShippedLate"))
{
// make the controller read-only by removing editing actions
NodeSet().SelectActions("New", "Edit", "Delete", "Duplicate", "Import")
.Delete();
// delete all remaining actions in the 'Grid' scope
NodeSet().SelectActionGroups("Grid")
.SelectActions()
.Delete();
// add new 'Navigate' action to the 'Grid' scope
NodeSet().SelectActionGroups("Grid")
.CreateAction(
"Navigate",
"Orders.aspx?OrderID={OrderID}&_controller=Orders" +
"&_commandName=Edit&_commandArgument=editForm1");
}
if (IsTagged("OrdersWaitingToShip"))
{
// sort grid1 and hide the 'ShippedDate' data field
NodeSet().SelectView("grid1")
.SetSortExpression("RequiredDate asc")
.SelectDataField("ShippedDate")
.Hide();
// add data field 'ShipCountry' to 'grid1' view
NodeSet().SelectView("grid1")
.CreateDataField("ShipCountry");
}
if (IsTagged("OrdersShippedLate"))
// sort and rearrange 'grid1' by placing ShippedDate, EmployeeID, and ShipCity first
NodeSet().SelectView("grid1")
.SetSortExpression("RequiredDate desc")
.ArrangeDataFields("ShippedDate", "EmployeeID", "ShipCity");
}
Visual Basic:
Protected Overrides Sub VirtualizeController(controllerName As String)
If (controllerName = "Orders" AndAlso IsTagged("OrdersWaitingToShip", "OrdersShippedLate")) Then
' make the controller read-only by removing editing actions
NodeSet().SelectActions("New", "Edit", "Delete", "Duplicate", "Import") _
.Delete()
' delete all remaining actions in the 'Grid' scope
NodeSet().SelectActionGroups("Grid") _
.SelectActions() _
.Delete()
' add new 'Navigate' action to the 'Grid' scope
NodeSet().SelectActionGroups("Grid") _
.CreateAction(
"Navigate",
"Orders.aspx?OrderID={OrderID}&_controller=Orders" +
"&_commandName=Edit&_commandArgument=editForm1")
End If
If (IsTagged("OrdersWaitingToShip")) Then
' sort grid1 and hide the 'ShippedDate' data field
NodeSet().SelectView("grid1") _
.SetSortExpression("RequiredDate asc") _
.SelectDataField("ShippedDate") _
.Hide()
' add data field 'ShipCountry' to 'grid1' view
NodeSet().SelectView("grid1") _
.CreateDataField("ShipCountry")
End If
If (IsTagged("OrdersShippedLate")) Then
' sort and rearrange 'grid1' by placing ShippedDate, EmployeeID, and ShipCity first
NodeSet().SelectView("grid1") _
.SetSortExpression("RequiredDate desc") _
.ArrangeDataFields("ShippedDate", "EmployeeID", "ShipCity")
End If
End Sub
This is the effect of the virtualization node sets evaluated at runtime.
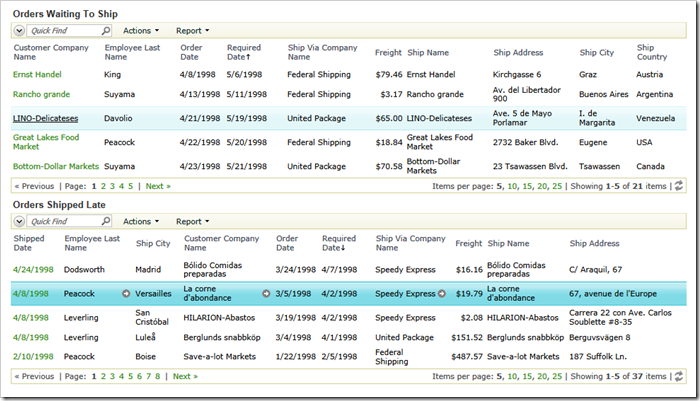